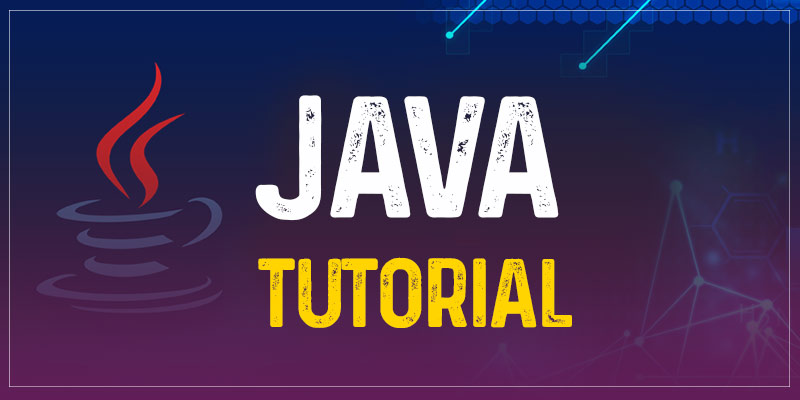
What is Java?
Java is a programming language and a computing platform developed by Sun Microsystems (present-day Oracle) in 1995 which was spearheaded by James Gosling. Java is a ‘high-level class-based object-oriented programming language’ that is used in a distributed environment. Java is easily readable and understandable and offers a higher level of abstraction.
There is a funny story as to how the name Java came into place. The programming language was named Oak initially because there was a big oak tree outside James Gosling’s office. It was later changed to Java because all the developers who were involved in the creation of this programming language drank coffee made using the Java bean variety from Indonesia.
The biggest jewel in the crown of Java is that it is ‘cross-platform which allows you to write the code in one platform and can run the code written in different setups’. Java allows you to write complete applications that can run on standalone computers and or in a distributed environment. You will need Java installed on your computer if you want several functions to run on the internet.
Java can be used to build web applications, standalone applications, mobile applications, and enterprise applications. Sun Microsystem released Java as open-source in 2006. In 2007 Sun Microsystems finished the process of making the core code of Java open source and free.
Above 3 billion devices/applications run on Java. Java has also continuously evolved over the years. With each update, new features were added to enhance the performance and the efficiency of the language.
Why Java?
The Java programming language is class-based, concurrent, and object-oriented. The basic principle of Java which ‘Write Once Read Anywhere (WORA)’ makes it more appealing to freshers as well as experienced workers. Over the years there has been only an increase in the number of programmers using Java to build applications in different domains.
The following pointers will throw light on the advantages of the Java programming language
- User friendly - Java is an easily readable language and offers different levels of abstraction for different users. Beginners can start with Core Java and after mastering it they can move towards Advanced Java.
- Platform Independence - Unlike the languages like C C++, Java code is once written can be run on machines with different Operating Systems like Linux, Windows, or Mac OS. This is because Java comes with a separate runtime environment JRE.
- Versatility - Java can be used to build web applications, android applications, and even in data science and machine learning applications. They offer high scalability and also provide concurrent solutions.
- Documentation - One of the major strengths of Java is its documentation. Since Java is an open-source language, you have access to a well-documented guide which you can refer to when you have to clarify doubts.
- Community support - Java has strong and robust community support and one can rely on the Java community to find help if they are in a programming rut.
- Powerful IDEs - Java has excellent IDEs like Eclipse, Netbeans, and IntelliJ which makes coding in java easy by a great deal. In addition to this, there are a vast number of automation tools and debuggers available.
- High performance - Just-in-time compiler in Java programming language enhances the execution of the code. This is possible because the compiler will compile a method block only when the method is called. This makes java a fast and time-efficient language.
Java - Overview
To gain complete knowledge about how a Java code is compiled and executed, the following terms should be familiarised as a prerequisite
JVM
Java Virtual Machine or JVM which acts as an engine that builds the runtime environment for running the Java code. JVM is the main reason that java can function as a cross-platform language. It helps in converting high-level code to bytecode. Each OS needs a different kind of JVM but the output of the JVM produced by different software will all be the same.
JRE
JRE or the Java Runtime Environment is software that runs on the operating system which makes the execution of Java applications possible. It accumulates the implementation of other core libraries and that of JVM. The JRE will consist of the components like deployment technologies, user interface toolkits, integration libraries, java database connectivity (JDBC), java native interface (JNI), Collections, Java archives (JAR), regular expressions, etc.
JDK
Java development kit or JDK is the software development environment that provides a set of tools and libraries to develop java applications and applets. If you are just interested in running a java program on your computer just installing JRE would suffice. But if you want to write java code and do actual programming, you have to install JDK. You can learn more in-depth about these topics in our Java course. You can check our Java syllabus.
Java - Environment setup
The environment setup for the Java programming language follows different steps for different operating systems.
To download and set up the local environment for Java programming language in your local Windows machine, the following steps are to be executed.
- Java SE is available for download at www.oracle.com. You have to create an account and download the SE that is suitable for your machine.
- Once you have downloaded the SE, you have to run the installer which installs both JDK and JRE.
- By default, the JDK is installed in the path "C:\Program Files\Java\jdk version where JDK version refers to the current update of the version of JDK installed.
- The directory in which the JDK is installed is called the JAVA_HOME.
- The path along with the bin of the JDK is called the Java classpath or simply PATH.
- Both these must be set as environment variables to enable the installation of directories.
- To set the environment variables, launch Control Panel -> System and security -> Systems -> Advanced System Settings on the left pane.
- Click on the environment variables button
- Under system variables, find the variable path and click on edit, and include the new Java path.
- Create a new system variable called JAVA_HOME and set the path up to the directory in which JDK is installed.
- This will enable your local windows machine to write and compile Java code. You can verify it by running the javac -version command on the terminal.
To download and set up Java on a Linux machine, the following steps are to be followed
- Create a directory in which JDK will be installed. The terminal commands are
$ cd /usr/opt
$ sudo mkdir java
- Extract the downloaded package in the java directory using the following commands
$ cd /usr/opt/java
$ sudo tar xzvf ~/Downloads/jdk-version-Linux-x64_bin.tar.gz
- Set the Ubuntu Machine for using the newly installed Java, by following the below commands.
$ ls -ld /usr/bin/java*
$ ls -ld /etc/alternatives/java*
Verify the installation using the following command
$ javac -version
In order to download and set up java in MacOS, the following steps are to be followed
- Navigate to www.oracle.com and login then accept the license agreement.
- Then look for the last stable update of JDK for the macOS and click on the Download button.
- Click on the disk image file and follow the instructions to install the JDK.
- Remove the disk image file.
- Now verify the installation with the javac -version command on the terminal
Java - Basic syntax
A Java program is a collection of objects which can be invoked by calling methods that have the definition for these objects. A Java program generally consists of the following parts. In this Java Programming Tutorial, let us go through the basic syntaxes that are used,
- Object - The object is also an instance of the class. It possesses both state and behavior which are defined by data members and methods respectively.
- Class - A class is a basic structure or blueprint which has a collection of objects with common behavior.
- Methods - A method is a Java Function that is used to alter the state of the data member and perform logical operations on it. A Class can have many methods that can be invoked by the respective call statements.
- Instance variables - The instance variables are also known as data members which are created by assigning a specific value to them. All the objects have a distinct set of instance variables.
The Rules to follow when writing the Java Program are given below
Case sensitive language - Java is a case sensitive language and it indicates that there is a difference when the same word is written in uppercase and lowercase. Proper attention should be paid to make sure the correct case is used or the compiler will throw a lot of errors.
Example: JAVA is different from java
Naming conventions - Java language follows a set of rules while naming the following
- Class name - The class name should always begin with an uppercase letter. If there are multiple words in a class name then the first letter of each word should be in uppercase.
Example: Animals, MyFirstJavaCode are all valid class names. - Method name - The method name should always begin with a lowercase letter. If there are multiple words in the method name then a camelcase naming convention should be followed.
Example: findArea(), display() are both valid method names - Variables - The variables can start with letters a-z or A-Z or with an underscore (_). It is preferable to avoid using single variables.
Example: _area, area, Area are all valid variable names. - Java file name - Java file name must match exactly with the class name of the program. When saving, the file name must be saved as class name.java. If there are multiple classes in the program the filename will be the same as the class name of the public class present in the program.
Example:SampleJavaProgram.java will be the file name when the class name is SampleJavaProgram
Objects and Classes
Java is an object-oriented programming language. Everything in java is denoted in terms of classes and objects with their respective attributes and behaviors.
Objects in Java
The object depicts the real-life entity. It is also an instance of the class. Typically an object consists of the following
- Identity - This gives an object a unique name and allows the object to interact with other objects.
- Behavior - This is the response of an object to the set of instructions (methods) invoked by the other objects.
- State - This reflects the properties of an object which are determined by the attributes and methods.
Declaring and initializing an object
When an object is created, an instance of the class is said to be created. All the objects share the same attributes and behavior of the class. But the state ( the value of these attributes and behaviors) is unique to each object. A class can have multiple objects.
An object is declared with the class name and the object name. An object with the given name will be created and assigned a null value.
An object is said to be initialized only when memory is allocated for the object with the new keyword. The new keyword also calls the constructor and the memory allocation happens for the object.
Classes in Java
A class is a user-defined prototype based on which objects are created. It defines the set of attributes and behavior that is common for all objects. Commonly a class will consist of the following components :
- Modifiers - A class should be in public or default access.
- Class keyword - The class keyword is used to create a class.
- Class name - The class name should begin with an uppercase letter.
- Superclass(optional) - The name of the class’s parent class. A class shall extend only one parent.
- Interfaces(optional) - A list of interfaces implemented by the class which is separated by a comma. A class can implement different interfaces at the same time.
- Body - The body of the class surrounded by curly braces { }.
Data fields define the attributes of the class and are usually in private access. Methods define the behavior of the class and generally have a public mode of access so that they can be called from the main method. Constructors are used for initializing the objects in the class and have public access in order to be invoked from the main method using the new keyword.
Constructors
Constructors in Java look similar to a method. But the constructors don't have a return type. Constructors are used to initializing an object and have a public mode of access generally. The constructors have the name as the name of the class.
Typically constructors are called when the object is initialized using the new() keyword. This makes sure that memory is allocated for the respective object and the attribute values are initialized. The constructor is invoked when the object is initialized or instantiated for the first time.
Rules for writing Constructors
- Constructors have the same name as the class name in which it resides.
- The constructors should not have a return type.
- Access modifiers can be specified to control access to the constructors.
Types of Constructors
- Default constructor or No argument constructor - As the name suggests the no-argument constructor has no parameters defined. If there is no constructor specified explicitly, then the compiler itself creates a default constructor that assigns values 0 and null, etc depending on the data type to the object which is being instantiated.
- Parameterised Constructor - A constructor that has parameters defined is called the parameterized constructor. If you want to define the values of the objects explicitly then a parameterized constructor should be used. If there are 2 constructors in the code, then the default constructor is invoked when parameters are not passed.
- Copy Constructor - A copy constructor is the one that initializes values of current the object using another object in the same class. A copy constructor is invoked when the object is passed as the argument to the function or when the object is copied to return it from the function. Java Training in Chennai at FITA Academy provides you extensive training of the Java Programming concepts with real-time examples and in-depth practice on code scripting under the mentorship of Expert Java Professionals.
Basic Data Types
The variables in java are used to hold values of different types. Based on the data type the operating system reserves memory space and decides what should be stored in the data type. Here in this Java Tutorial for Beginners let us see some of the Basics Data types.
The Data type is further classified into two groups and they are
- Primitive Data Types - int, short, byte, float, char, boolean, and double
- Non Primitive Data Types - Arrays, Classes, and Strings
The eight various primitive data types in Java are,
Data Type |
Size |
Description |
short |
2 byte |
It stores the complete whole numbers from -32,768 to 32,767 |
byte |
1 byte |
This stores the whole numbers from -128 to 127 |
long |
8 bytes |
It stacks the whole numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. |
double |
8 bytes |
It hoards the fractional numbers. This is more than enough to store 15 decimal digits. |
int |
4 bytes |
It piles the whole numbers that range between 2,147,483,648 - 2,147,483,647 |
float |
4 bytes |
This stacks the fractional numbers that are sufficient enough for storing 6 to 7 decimal digits. |
char |
2 bytes |
It stacks a single letter/ character or the ASCII values. |
boolean |
1 bit |
This stores the true or false value. |
Numbers
The Primitive Number types are further divided into two different groups and they are
- Integer types - This stores positive, negative, or whole numbers (such as 123 or -456), without any decimals. The Valid categories are short, long, int, and byte.
- Floating Point Types - It interprets the numbers that are within the fractional part that consists of more than one decimals. The two varieties are float and double.
Types of Integer
Byte
A Byte Data type stacks the whole numbers which range from -128 to 127. This can be used in the position of the other integer types and int to save the memory when you are confident that the values are within the limit -128 and 127
Int
An int data type is capable of storing the complete whole numbers from the - -2147483648 to 2147483647. Usually, an int data type is the commonly chosen data type if the created variables are within the numeric value.
Short
A Short Data type hoards the Whole Numbers which ranges from 32768 to 32767:
Long
Long Data type shall hoard the whole numbers that range from -9223372036854775808 to 9223372036854775807. It is utilized when an int is not larger for storing the value. Important Note: You must end a value with "L":
Floating Point types
You can use the Floating-point type when you require the number that is with a decimal, for instance, 9.99 or 3.14515.
Float
A Float Data type stacks the fractional number that ranges from 3.4e−038 to 3.4e+038. Important Note: You must end the value with "f":
Scientific Numbers
There are possibilities that the floating-point number shall also be the scientific number along with an "e" denotes the power of 10:
Booleans
The Boolean Data types are termed with a Boolean keyword and it takes values of true or false:
A boolean data type is declared with the boolean keyword and can only take the values true or false:
Java Notes for beginners
The Boolean values are generally used in conditional testing
Characters
A char data type is primarily used for accumulating the characters that are single. These characters should mostly be encircled by single quotes such as 'A' or 'D'
Strings
A String Data type is utilized for storing the sequence of the characters (text). The String values should always be surrounded by double-quotes
Non-Primitive Data Types
These Data types are also called the reference types since they refer to the objects.
The distinction between primitive & non-primitive data types,
- A Primitive type is the predefined one in the Java language. A Non- primitive type is developed by the programmer and it is not illustrated by Java.
- Usually, a primitive type consists of values, whereas the non-primitive kind begins with the uppercase letters.
- The Non-primitive types are applied in the call methods for performing a few operations, however, the primitive types cannot.
- The Primitive types begin with the lowercase letter, whereas the non-primitive kinds begin with an uppercase letter.
- The Capacity of the primitive type relies on data type, whereas the non-primitive type consists of a similar size
Java Variables
The variable aids us with named storage which the programs can manipulate. All the variables in Java comprise a specific type and it resolves the layouts and size of the variable memory & the array of values that are stored in a memory along with the set of operations that is applied to the variable.
It is mandatory to declare the variables prior to using it. Mentioned below are the basic form of the variable declaration -
The Data type is Java's Data Types and it is the variable in the name of a variable. For declaring the above one plus variable of the mentioned type, you can make use of the comma-separated list.
Below are the valid example of the variables that are used for declaring and initializing Java-
For Example
In this Java Basic Tutorial, we will explain to you different variables that are available in Java and the three different types of variable are
- Local variables
- Instance variables
- Class/Static variables
Local Variables
- A Local variable is usually declared in block, methods, or constructors.
- The Local Variables are developed when the constructor, methods, or the block is entered and the variable is eliminated once when it steps out from the block, constructor, and method.
- The Access Modifiers cannot be applied for the local variables.
- A Local Variable is deployed at the stack level internally.
- The Local variable is visible only if it is within a declared block, method, or constructor.
- You will not have the default values for the local variables, and the local variables should be declared and the initial values should be allowed prior to using it.
Example
The Age is the local variable. It is defined inside the cubAge() method and the scope of it is limited only within this method.
To use age without initializing, so that it would be given as Error during the time of compilation.
Instance Variable
- The Instance Variable is declared in the class, however outside the block, method, or constructor.
- If space is allowed for the objects in the heaps, the slot for every instance variable value is built.
- An Instance variable is developed when the object is developed with the application of the keyword 'new' and it is destroyed if the object is destroyed.
- The Access Modifiers are provided for the instance variables.
- The Instance Variables are declared when the class level after or before use.
- An Instance variable is visible for all the methods, blocks, and constructors in a class. Usually, this is recommended to make these variables private for the access level. Yet, the visibility for the Subclasses is provided for the variables with the utilization of the access modifiers.
- The Instance variables do have default values. For Boolean, it is either false, for Number, it is 0, and for the object references, it is null. The values shall be assigned at the time of declaration or within a constructor.
- An Instance variable is accessed straightly by calling the variable name inside a class. And within the static method, it must be termed using a fully qualified name. ObjectReference.VariableName.
Static/ Class Variables
- Over here you will have exactly one copy of all the class variables for a specific class, irrespective of how many objects are developed from it.
- The Static Variables are used rarely apart from declaring them as the constants. The Variables and Constants are termed as private/public, static, and final. The Constant variables do not change right from the beginning of a value.
- A Class Variable is also called the Static Variables and this is declared along with a Static keyword in the class, however, outside the block, method, or constructor.
- The Static Variables are stacked in the Static Memory. A Static Variable is used for building the program right from the beginning and it is terminated as soon as the program stops.
- The Visibility is more familiar for the instance variables. Yet, the most static variables are termed public as they are found for the users of a class.
- The Default values are similar to that of the instance variables. For Booleans - it is false, for object references - this is null, and for numbers - it is 0.
- Mostly the values are assigned while the declaration or within a constructor. Further, the values are assigned to the special static initializer blocks.
- The Static Variables shall be pervaded while calling a class name ClassName.VariableName.
- While declaring the class variable as the Public Static final, then the variable names i.e the constants everything is used with the upper case.
- When the Static Variables don't seem to be final and public, then the naming syntax is similar to that of the local and instance variables.
Modifier Types
A Modifier is a keyword that you can add to the definition of changing the meanings. The Java language consists of a broad range of modifiers, which includes the following,
- Java Access Modifiers
- Non Access Modifiers
You can use the modifier that includes the keywords, definition of the method, variable, or class.
Access Control Modifiers
Java enables you with more access modifiers for setting the access levels to the variables, methods, constructors, and classes. The four different access levels include
- Noticeable only to the Class (private)
- Noticeable to the package and the default also no modifiers are required.
- Noticeable to all the subclasses and packages.
- Noticeable to World (public)
Non- Access Modifiers
- Java supports you with a number of non-access modifiers for achieving other functionalities.
- A Final Modifier is used in finalizing the performance of methods, classes, and variables.
- A Static Modifier is utilized in developing the class variables and methods.
- The Volatile and Synchronized Modifiers are used in threads.
- An Abstract Modifier is used for building abstract methods & classes.
Java Operators
Java provides a wide range of operators for manipulating the variables. In the given below example, you can make use of the + operator for adding together two values,
For Instance,
Though an operator is commonly used for adding together the two values as per the example given above, you can use it for adding together the variable and the value, or the variables and the other variable.
In this Tutorials on Java, let us see the different types of operators that are used,
- Arithmetic operators
- Comparison operators
- Assignment operators
- Bitwise operators
- Logical operators
Arithmetic operators
The Arithmetic operators are predominantly used for performing general mathematical operations.
Operator |
Description |
Example |
- (Subtract) |
It subtracts one value from the other |
x+y |
* (Multiplication) |
It multiplies two values |
x*y |
+ (Addition) |
It adds two or more values together |
x+y |
% (Modulus) |
It sends back the division remainder |
x%y |
/ (Division) |
It divides one value by another |
x/y |
+ + (Increment) |
It increases the value of the variable by 1 |
++x |
-- x (Decrement) |
It decreases the value of the variable by 1 |
--x |
Comparison Operators
The Comparison Operators are used in comparing values
Operator Name |
Description |
Example |
== |
Equal to |
x==y |
> |
Greater than |
x > y |
< |
Less than |
x |
!= |
Not equal |
x != y |
<= |
Lesser than or equal to |
x <=y |
>= |
Greater than or equal to |
x >= y |
Assignment operators
The Assignment operators are used for assigning the values to the variables. From the below example, you can use the Assignment Operator (=) for assigning the value 75 to variable x:
For Example
The groups of Assignment operators include
Operators |
Example |
Result |
+= |
X + = 8 |
X = x+8 |
-= |
X - = 9 |
X = x-9 |
= |
X = 5 |
X =5 |
/ = |
X / =6 |
X + X/6 |
*= |
x* =4 |
X = X* 4 |
&= |
X & =7 |
X = X& 7 |
%= |
X% = 2 |
X= X%2 |
^= |
x ^=3 |
X = x ^=3 |
|= |
X |=5 |
X = x|5 |
>>= |
X >> =1 |
X = x>>1 |
<<=4 |
X << =4 |
X = x<<4 |
Bitwise operators
The Bitwise Operators are used in executing the Binary logic with bits of the long integer or the integer.
Operator Name |
Description |
Example |
Similarity |
Result |
Decimal |
| (or) |
It sets all the bits to 1 when any of the bits is 1 |
5|1 |
0101| 0001 |
0101 |
5 |
& (And) |
It sets all the bits to 1 when both the bits are 1 |
5&1 |
0101 & 0001 |
0001 |
1 |
~ (Not) |
It inverts all the bits |
~5 |
~0101 |
0101 |
5 |
<<(Zero fill, left shift) |
It shifts the left by moving the zeroes from the right towards the left. |
7<<1 |
1001<<1 |
0010 |
2 |
^ (XOR) |
This set all the bit to 1only when one of the 2 bits is 1 |
4^1 |
0101 ^ 0001 |
0100 |
4 |
>>> (Zero fill, right shift) |
It shifts right by moving the zeros that are in the left and permits the bits that are in rightmost to fall |
9>>>1 |
1001 >>>1 |
0100 |
4 |
>> (Signed Right Shift) |
This shifts the right by moving the zeroes from the left and allowing the rightmost bit to fall |
8>>1 |
1001>>1 |
style="text-align: center;" 1100 |
12 |
Logical operators
The Logic Operators are used for identifying the logic between values or variables.
Name |
Operator |
Description |
Example |
! |
Logical Not |
It reverses the result and it returns the false in case the results are true. |
!(x<5 && x 10) |
|| |
Logical Or |
It refutes true when one of the statements is true. |
x<5||x<4 |
&& |
Logical and |
It returns to true when both the statements are true |
x<7&& x<10 |
Loop Control
There may come a few circumstances where you should execute the block of code numerous times. Generally, the statements will be executed in consecutive order. Like, the first statement will be executed first, then the next by second, and so on.
The Programming languages offer different control structures that permit you for the most complex execution paths.
In general, the Loop Statements permits us to execute a particular statement or a group of statements at different times, and given below is the common form of loop statement that is used in most programming languages.
There are three types of Loops in Java and, they are
- for loop
- while loop
- do-while loop
for loop
The for loop in Java is used for iterating a specific factor of the program manifold times. Supposing the total number of iterations is fixed, then it is advisable to use for loop statements.
Syntax
The Syntax of a for loop is-
Given below is the flow of Control in the for loop:
- Usually, the initialization step is executed first, and it is performed only once. This step permits you to initialize and declare the loop control variables and usually, these steps complete with a semicolon (;)
- Later, the Boolean Expression is calculated regularly. After that, if it is true, then the body of a loop needs to be executed. In case if it turns out to be False, then the body of the loop shall not be executed along with the control jumps for the next statement past a for loop statement.
- The body of a for loop is executed, then the control bouncebacks in updating the statement. A for loop statement permits you to update the loop control variables. Also, it is possible that this statement shall be left blank with a semicolon in the end.
- A Boolean expression is estimated again. In case, if it is true, then the loop executes and repeats the process of the body of a loop; later this step is updated and also the Boolean Expression. Once the Boolean expression is concluded to be False, then the for loop statement terminates itself.
While Loop
The while loop statement frequently executes the target statement in the Java Programming language until the conditions that are provided are true.
Syntax
The Syntax of a While Loop is-
Over here, statements(s) shall be either a single or block of statements.A condition can be any expression and the true will be a non-zero value.
While executing, supposedly the boolean-expression outcome is true, then the inside actions of the loop can be executed. And this will prolong until the expression returns to true.
Meanwhile, the conditions turn out to be false, then the program control shall pass it to the line automatically succeeding a loop.
The important point of a While loop is that a loop may not run always. If the expression is tested and if you get the result as false, then the loop of the body shall be skipped and the very first statement after the while loop shall be executed.
do...while loop
The do...while loop is identical to a while loop, except if a do...while loop is assured for executing at least once.
Syntax
Following is The Syntax of a do... While Loop-
Check whether the Boolean expression occurs at the end of a loop, then the statement in a loop executed before a Boolean is tested. When the Boolean expression is true, then the control bounces back to the do statement and the statement in a loop is executed once again. This process is repeated further until the Boolean expression results in false.
Loop Control Statements
A Loop control statement modifies the execution from the normal sequence. In case the execution flees a scope, then the automatic objects which were created in that particular scope are destroyed.
A Loop control statement modifies the execution from the normal sequence. In case the execution flees a scope, then the automatic objects which were created in that particular scope are destroyed.
In this Java Tutorials for Beginners we have discussed the different Control statements supported by Java are as follows
- continue statement
- break statement
- continue statement
- In the for loop, the continue keyword induce control automatically and moves to the update statement.
- In do/while loop or while loop, it controls automatically and dives to a Boolean expression.
A Continue keyword is used in any loop control structures. This causes a loop to jump fastly to the other iteration that is in the line of the loop.
Syntax
Break Statement
A break statement in the Java Programming language is used for two different purpose
- In case, the break statement is confronted inside a loop, then the loop is instantly terminated, and now the program control proceeds with the next statement that follows the loop.
- It can be used for terminating in case of switching the statement
Syntax
Java Online Course at FITA Academy provides in-depth training of the Java programming concepts with complete hands-on training and real-time practices under the guidance of Expert Java professionals.
Decision Making
A Decision-Making structure includes one or more than one condition for evaluating or testing the program with the statement or the statements that shall be executed when the condition is identified as true, and in addition to that, the other statements can be executed when the condition is identified as false.
Below is the general method of typical decision-making structure which is found in predominant programming languages.
The Java Programming language supports us with the following types of Decision-making statements.
- if...statement
- nested if statement
- if...else statements
- switch statement
if...statement
The if statement encompasses the Boolean expression that is followed by more than one statement.
Syntax
When the Boolean Expression is assessed to be true, then a block of code which is inside the if statement is executed. In case if it is not, then the first group of code after the end of an if statement shall be executed (on closing the curly braces)
nested if statement
It is permissible to nest if-else statements i.e you can make use of else if or one if statement inside the other else if or if statement.
Syntax
if....else statement
The 'if statement' is superseded by the optional else statement, that executes while a Boolean expression is false.
Syntax
Whenever the boolean expression is assessed to true, then supposing the block of code is executed, contrarily the else block of code shall also be executed.
An if...else if...statement
The if statement is succeeded by the optional else if...else statement, that is useful in testing different conditions using the single if.. else if statement.
- While using the if, else if statement there are few things that must be kept in the mind.
- An if shall have one or zero else's and it should come after the any else if's
- The if can have zero for many else if's and it should come before an else.
- If an else is succeeded, then none of the rest else's or the else if's would be tested.
Syntax
Switch Statement
The Switch Statement permits the variables to be tested for equal opportunity against the set of values. All Values are called the case, and the variable that is going to be switched on is verified for each case.
Rules that are applied to the Switch Statement
- A Variable is used in the Switch statement that can be strings, integers, enums, and Convertible integers like (byte, char, short)
- The value for the case should be the same as the data type just like a variable in the switch and it should be literal or a constant.
- It is possible that one can have more case statements within the switch. All the case is followed by a value that can be compared to and to the colon.
- In case the break statement is reached, then it's switch terminates the control flow hops to next line that supersedes a switch statement
- In case when a variable is switched to the equal case and the statements which follow the case would execute continuously till the break statement is being reached.
- The Switch statement shall consist of an optional default case, that should appear at the end of a switch. A default case could be used in performing the task when no single case is true. The break is not required in a default case.
- It is not mandatory that all the cases are required to have a break. If at all there are no break cases found, then the flow of control falls through the subsequent cases till the break is reached.
For Example
Output
Java Numbers
Usually, when you work with Numbers, you can use the primitive data types namely long, double, byte, and int.
Anyhow, in the development, you can come across circumstances where you must use the objects rather than primitive data types. To reach this, Java provides the Wrapper Classes.
The Wrapper Classes ( Long, Byte, Double, Float, Integer, and Short) are the different subclasses of an abstract class number.
An Object is the Wrapper Class that consists of Wraps and its corresponding primitive data types. For converting the primitive data types to objects it is called boxing and it is to be looked after by a compiler.
Hence, while using the Wrapper class you should pass the value of a primitive data type for the constructor of a Wrapper Class.
Now, the Wrapper Object shall be converted to a primitive data type and this practice is known as unboxing. The Number Class is a unit of java.lang package.
The different types of Numbers in Java are
When b is designated as the integer value, and now the compiler boxes the integer as b is the integer object. Further, b is now unboxed so it can be added as an integer.
Number Methods
In this Java Tutorial for Beginners let us go through the instance methods and all the subclasses which a Number class implements
Methods |
Description |
compareT0 () |
A compare() method is used for comparing a Number Object that calls the method to an argument. It is probable to compare the Long, Byte, and Integer. Note: It can't compare two different types, both the Number object and the arguments that use the invoke method must be of a similar kind. |
xxxValue () |
This method helps in converting the value of a Number object that calls a method to a primitive data type and this is a retreat method. |
valueOf() |
It sends back the integer object that holds the value of a specific primitive. |
equals() |
This method is used for arbitrating whether the total number of objects that invokes an equal method to that of the object which is passed as arguments. |
parselnt() |
T he parselnt() method is used for getting a primitive data type that is certain to the string. A parseXxx() is the static method that consists of one or two arguments. |
tostring() |
This Returns the String Object by representing a value of the mentioned Integer or int. |
min() |
It sends back the smaller one of the two arguments |
max() |
This method provides a maximum of two arguments. An Argument can be long, double, float, and int. |
exp() |
It sends the base of Natural logarithms, e, to the power of an argument. |
pow() |
It helps in returning the value of the first argument and that it is raised to the power of the 2nd argument. |
Java Characters
Usually, whenever you work with the characters, you can use the data types char.
For Example
In the development, you can come across the situation where you should use the objects rather than the primitive data types. To reach this, Java gives the wrapper class character of a primitive data type char. This character class provides a more useful class i.e (static methods) to manipulate the characters. Then, you can build the Character object within a Character constructor.
Character ch = new Character('a');
A Java Compiler can create the Characters of the Object for you in certain circumstances. For instance, in case if you pass the primitive char into the method that seeks an object, then the compiler immediately converts the char to the Character for you. It is called autoboxing or unboxing in case the conversion turns the other way.
Escape Sequences
The character that is anticipated by the backslash (\) is the escape sequence and it consists of unique meaning to a compiler.
The below table clearly depicts the Java Escape sequences:
Escape Sequence |
Description |
\t |
It helps in inserting the tab during the escape sequence. |
\n |
It aids in inserting the Newline to the text during the escape sequence. |
\f |
It helps in inserting a form feed in a text during the escape sequence. |
\b |
It aids in inserting the backspace in the text during an escape sequence. |
\r |
This inserts the carriage return in a text during this escape sequence. |
\' |
This inserts a single quote character in the text while the escape sequence. |
\\ |
It helps in inserting the backslash character in a text while the escape sequence. |
\" |
This helps in inserting the double-quote character in a text at the time of escape sequence. |
The different Character Methods are:
Methods |
Description | |
isDigit() |
This helps in determining whether the specific char value is a digit |
|
isLetter() |
It helps in finding whether the mentioned char value is the letter. | |
|
This method helps in returning the |
|
isUppercase() |
This method helps in returning the uppercase form of a specified char value. |
|
isWhitespace() |
This method identifies whether a specified char value is the White space that consists of tab, newline, or space. |
|
isLowercase() |
It is used for identifying in case the specified char value is lowercase. |
|
toLowercase() |
This helps in returning the lowercase form to the mentioned char value. |
|
toString() |
It sends back the String Object which interprets the mentioned character values that are one-character strings. |
|
toUppercase() |
This method sends the Uppercase form of the described char value. |
Java Training in Bangalore at FITA Academy provides comprehensive training in the Java programming language from the basics to the advanced topics under the guidance of Expert Java Professionals from the programming industry.
Java Strings
The Strings are used for stacking shorter text.
The String Variable consists of the compilation of characters that are bounded by the double quotes:
Example
We can build the variable type of string and assign them to the value
Create the variable of type String and assign it a value:
String Length
The String Length in Java is the object that consists of the methods which can exhibit operations on the strings. For instance, the length of the string is found with a length() method:
More String Methods
More string methods are found and they are toLowerCase() and toUpperCase().
To identify a Character in the String
An indexOf() method send back the index (the exact position) of the first occurrence of the described text in the string (inclusive of the whitespace):
Java Notes for Beginners:
Java usually counts a position from zero So,
- 0 is the 1st position
- 1 is the 2nd position
- 2 is the 3rd position and so on...
String Concatenation
The Operators could be used between the strings for combining them and it is called concatenation.
Java Notes for Beginners:
We have inserted an empty text (" ") for developing the space between the First & Last Name on the print.
Further, you can also use a contact() method for concentrating the two strings
Special Characters
As it is important that the strings should be written within a quote since Java would misinterpret this as the string and provoke the error:
The best solution for avoiding a problem is by using a backslash escape character.
A backslash (\) escape character help in modifying the special characters into the string characters:
Escape Character |
Result |
Description |
\" |
" |
Double Quote |
\' |
‘ |
Single Quote |
\\ |
\ |
Backslash |
A sequence \" adds the double quote to the string:
The sequence \' aids in adding the single quote in the string
The sequence \\ helps in inserting the single backslash to a string:
This \\ sequence adds a single backslash to a string:
This \\ sequence adds a single backslash to a string:
Java Notes for Beginners:
It utilizes the + operator for concatenation & addition. The Numbers are added and the strings are concatenated.
When you add to numbers, the outcome will be a number
When you add the 2 strings, the outcome will be the string concatenation:
Java Arrays
Generally, Java enables a Data Structure, an array, that stacks in the fixed-size of the consecutive elements of similar kinds. The Arrays are used for storing the acquisition of data and it is useful to consider that an array as the compilation of variables of the same types. Rather than declaring the individual variables like number0, number99.... and so on, you can declare 1 array variable like numbers and utilize number[0], a number[99]... and so to interpret the individual variables.
In this Java Programming Tutorial, we will make you understand how to create the arrays, declare the array variables, and the process of arrays that are used in indexing the variables.
For creating an Array
It is possible to create the array using a new operator by following the syntax-
Syntax
The above statement helps in two things
Creates the array utilizing a new dataType[arraySize] It designates the reference of a newly created array and the variable of the arrayRefVar.
You can create, declare, and assign the reference of an array to a variable and can be merged into one statement as given below
Processing Arrays
At the time of Processing the array elements, you can either use the foreach or a for a loop as all the elements in the array are of a similar type and the size of an array is known.
The Foreach Loop
JDK 1.5 popularized the new for loop that is known as enhanced for loop or foreach loop that allows you to pass through the entire array orderly without using the index variable.
Passing the Arrays to the Methods
Just like how you pass on the primitive type of values to the methods, also you could pass the arrays to the methods.
You can call the above by passing the array. For instance, the following statement calls the print array method for displaying 5,7,2,8,9 and 2-
Java Date and Time
Java supports the Date class that is available in java. util package, this class encloses the current time and date.
A Date Class hold two constructors as given below
Constructor |
Description |
Date () |
The Date() Constructor loads the object with the current date & time. |
Date (long millisec) |
The Date (long millisec) accepts the argument that balances the total number of a millisecond that has run out since the midnight of Jan 1, 1970. |
Below are the methods of Data Class
Method |
Description |
boolean before (Date date) |
It rebounds to true when the requested Date object consists of a date that precedes the one mentioned date, or otherwise, it rebounds to false. |
boolean after (Date date) |
It rebounds to true when the requested Date object consists of a date that is next to the mentioned date, or otherwise, it returns false. |
Object clone () |
It duplicates the requested Date Object. |
int compareTo (Object obj) |
It operates uniformly to compareTo(Date) when the obj is of the class date. Or else it delivers the ClassCast Exception. |
int compareTo(Date date) |
|
int compareTo(Date date) |
|
long getTime() |
It sends back the number of milliseconds that have passed through since January 1, 1970. |
String String() |
It converts the requested Date object to a string and it sends back the result. |
void setTime (long time) |
It sets the date and time as mentioned by time, that depicts the lapsed time in the milliseconds from the midnight of January 1, 1970. |
int hash code () |
It rebounds the hash code for the requested object. |
Get the Current Date & Time
It is the easiest method for accessing the current Date & Time in Java. You can make use of the simple Date object along with the toString() method for printing the current date & time as given below:
Date Comparison
Mentioned below are the three different types for comparing two dates
- You can use the following methods before (), equals(), and after(). As the 12th month comes prior to the 18th for instance the new date ( 95, 3,12). Before (new date 95,3,18) returns true.
- You can also use the getTime() for knowing the total number of milliseconds that have passed by since January 1, 1970 midnight for both the objects and you can compare these values.
- You can also use the compareTo() form, which is characterized by the implemented Date and Comparable interface.
Date Formatting Using a SimpleDate Format
The SimpleDateFormat is the concrete class that is applied for parsing and formatting the dates in a locale-sensitive manner. A SimpleDateFormat permits you to begin by selecting any user-defined patterns for the date-time formatting.
Time DateFormat Codes
If you need to specify a time format, then you can use the time pattern string. When using this template, all the ASCII letters are reserved as the pattern letters and it is termed as following:
Character |
Description |
Example |
y; |
It depict the year |
2021 |
d |
It represent the day in the month |
3 |
G |
It represent the Era designator |
AD/BC |
M |
It indicates the month in a year |
August or 08 |
h |
It represent the hour from AM to PM (1-12) |
12 |
m |
It represents the Minute in the hour |
30 |
H |
It depicts the hours in a day (0-23) |
22 |
s |
It represents the second in time |
44 |
S |
It depicts the Millisecond in a day |
234 |
D |
It interprets the days in a year |
365 |
E |
It represents the days in Week |
Monday |
F |
It depicts the day of Week in a month |
3rd (Third Sunday in April) |
W |
It represents the Week in a month. |
2 |
w |
It interprets the week in a year |
34 |
a |
It is the AM/PM marker |
PM |
K |
It indicates the hours in AM/PM (0-11) |
8 |
k |
It depicts the hours in a day (1-24) |
17 |
‘ |
Escape for Text |
Delimiter |
z |
It indicates the Time Zone |
Eastern Standard Time |
‘‘ |
Single Quote |
‘ |
Date Formatting Using a SimpleDate Format
The SimpleDateFormat is the concrete class that is applied for parsing and formatting the dates in a locale-sensitive manner. A SimpleDateFormat permits you to begin by selecting any user-defined patterns for the date-time formatting.
Time DateFormat Codes
If you need to specify a time format, then you can use the time pattern string. When using this template, all the ASCII letters are reserved as the pattern letters and it is termed as following:
Date Formatting Using the printf
Time and Date formatting is performed easily using a printf method. You can make use of the two-letter format and begin with the t and it ends in one of the letters of a table that are shown in the code below.
Java Notes for Beginners
It is not advisable to supply the date numerous times to format all the parts. So, you can use the format string that indicates an index of an argument that should be formatted. On doing that the index should automatically follow the % and it should be eliminated by a $.
Time and Date Conversion Characters
Character |
Description |
Example |
F |
It is the ISO 8601 Date |
2004-03-2009 |
D |
It is the US Formatted Date(mm/day/year) |
03/11/2021 |
c |
It is used for representing the complete Date and Time |
TUE Mar 21 08:23:34 CDT 2009 |
T |
It depicts 24-hour time |
17: 07:21 |
r |
It represents the 12-hour time |
07:08:22 PM |
Y |
It is used for representing the Four- Digit year( with the leading zeros) |
2006 |
C |
It represents the first 2 digits of a year( with the leading zeros) |
20 |
y |
It depicts the last 2 digits of a year (with the leading zeros) |
06 |
B |
It is used for representing the Full Month name |
March |
m |
It is used for interpreting the Two-digit month(with the leading zeros) |
03 |
b |
It used for representing the abbreviated month name |
Mar |
d |
It is used for representing the two-digit day (with the leading zeros) |
05 |
A |
It used for depicting the Full Weekend Name |
Tuesday |
e; |
It used for interpreting the Two-digit days (without the leading zeros) |
8 |
j |
It represents the three digit day of the year( with Leading zeros) |
069 |
k |
It is a Two-digit hour i,.e between 00 and 23(without leading zeros) |
18 |
| |
It is the Two-digit hour between 1 to 12 (without any leading zeros ) |
6 |
| |
It is the Two-digit hour between 01 to 12(along with the leading zeros) |
06 |
S |
It is the Two-digit seconds (with leading zeros) |
19 |
N |
It is the Nine-digit nanoseconds (along with the leading zeros) |
0560000 |
P |
It is the Upper case or afternoon maker |
PM |
Z |
It Indicates the time zone |
PST |
Java Training in Hyderabad at FITA Academy provides you holistic training of the Java programming language from its fundamentals to the complex topics under the guidance of real-time Java professionals.
Java Regular Expression
The Regular Expressions are a unique sequence of characters that helps you to find or match the set of strings, or other strings, upon using a specialized syntax that is held in the pattern. It can be used for editing, searching, or for manipulating the text or data. In this Java Tutorial for beginnerslet us see in detail the Regular Expressions that are used in Java.
Usually, the java. util. regex pack comprises of three classes and they are
- Pattern Class
- PatternSyntax Exception
- Matcher Class
- Pattern Class - The Pattern Objects is an organized interpretation of regular expressions. A Pattern Class usually gives no Public Constructors. For creating the pattern, initially, you should call one of the public static compile() methods that send back the Pattern object. These methods usually accept a regular expression as a first argument.
- PatternSyntax Exception - These are the objects that are the unchecked exception that notifies the syntax error on the regular on regular expression pattern.
- Matcher Class - The Matcher Objects are the engine that represents the patterns and it executes the match operations counter to the input string. Likewise a Pattern Class, the Matcher illustrates the no public constructors. You can gain the Matcher Object by calling the matcher() method on the Pattern object.
Capturing Groups
This is one of the ways of treating different characters as one unit. It is created by placing characters that should be organized inside the set of parentheses. For instance, a regular expression (Apple) creates one group that contains the letters "A", "P", "P", "L", and "E". Capturing the groups is counted by the opening parentheses from left to the right side.
In these expressions ( (A) (B(C))), for instance,
- ((A)(B(C)))
- (A)
- (B(C))
- (C)
Identifying the groups that are available in these expressions are called a group count method on the matcher object. A group count method sends back the int that displays the total number of captures that the group presents in a matcher's pattern.
Also, there is a special group, group 0, that always interprets the complete expression. And this group will not be accommodated in the total report of the groupCount.
Syntax of Regular Expression
Sub Expression |
Matches |
$ |
It helps in matching the end of lines |
^ |
It aids in matching the beginning of a line |
[...] |
It helps in matching the single characters in the bracket. |
[^...] |
It supports matching any single characters that are not in brackets. |
\Z |
It is the end of the complete string except for the permitted end line terminator. |
\z |
This is the end of the entire string. |
\A |
It is the starting of the complete string. |
re+ |
It helps in Matching 1 or more previous things. |
re? |
It aids in matching 0 or 1 occurrences to the preceding expression. |
re* |
It helps in matching the 0 or more occurrences to the foregoing expression. |
re{n} |
It literally matches the n number of occurrences of the foregoing expression. |
re{n,m} |
It helps in matching at least one n and most of the ‘m’ occurences of the foregoing expression. |
re{n,} |
It helps in matching n or more number of occurrences of the leading expression. |
(re) |
It helps in Grouping regular expressions and it helps in remembering the matched text. |
a|b |
It helps in matching either a or b |
(?: re) |
It aids in grouping the regular expression without the memory of matched text. |
(?> re) |
It supports matching the independent pattern without any backtracking. |
\W |
It helps in matching the nonword characters. |
\w |
It aids in matching the word character. |
\D |
It supports in Matching the non-digits |
\d |
It helps in matching the Digits That is equivalent to [0-9] |
\S |
It aids in matching the non-white space |
\s |
It helps in matching the white space which is equivalent to [t\n\r\f] |
\A |
It supports matching the starting of a string. |
\Z |
It supports matching the end of a string and in case if a newline is found then it matches prior to the new line. |
\z |
It helps in matching to the end of a string. |
\n |
It is the back-reference for capturing the group number ‘n’ |
\G |
It matches to the point where the last match is finished |
\B |
It aids in matching the non-word boundaries |
\b |
It helps in matching the word boundaries that are outside a bracket. It helps in matching the backspace (0*08) when it is inside the bracket. |
\D |
This Escapes all the characters that are up to \E |
\E |
It helps in ending the quoting that begins with \Q |
\n\t etc |
It aids in matching the newline and the carriage return tab. |
Different Methods of a Match Classer
The Index methods give us resourceful index values that showcase the exact match where it is found in an input string.
- public int start) - It helps in sending back the start index of a previous match.
- public int end() - It refutes the offset only after when the last characters are matched.
- public int start(int group) - This sends back the beginning of an index that is laterally captured to the given group at the preceding match operations.
- public int end(int group) - It sends back the offset of the last character of concatenation and it is clocked by the set that is given at the older match operation.
Study Methods
A Study method reviews an input string and it sends back the Boolean that points out whether the pattern is found or not. Some of the common study methods are listed below -
- public boolean find() - It helps in identifying the progression of an input sequence that adheres to the pattern.
- public boolean lookingAt() - It tries to match the complete region against a pattern.
- public boolean matches() - It tries to match the complete region against a pattern.
- public boolean find (int start) - It helps in resetting the matcher and then it tries to identify the concatenation of input sequence that coheres to the pattern, that begins at the beginning of a specific index.
PatternSyntax Exception Class Methods
This is an unchecked exception that denotes the syntax error on the regular expression pattern. A PatternSyntaxException class gives the below methods for identifying where it went wrong
- public int getIndex () - It restores the error index
- public String getMessage () - This sends back the multi-line string that consists of a description of a syntax error along with its index. Also, it sends back the erroneous regular expression pattern and the visual indication of an error-inside within a pattern.
- public String getDescription () - It helps in reclaiming the description of the error.
- public String getPattern () - It restores the erroneous usual expression pattern.
Java Methods
It is the compilation of statements that are organized together for performing the operations. You can call the System.out.println() method, for instance, the system can execute different statements for displaying the message on the console.
In this Java Fundamental Tutorial, you will comprehend how to create your methods with or without returning the values for invoking a method and to apply the method of abstraction to the program design.
For creating the Method
Consider the below example
Syntax
Over here,
- int - return type
- public static - modifier
- methodName - It is the name of a method
- int a, int b - It is the list of parameters
- a, b - It is the formal parameters
A Method definition consists of a method body and method header.
Syntax
The above syntax also includes:
- returnType - This method shall return the value
- modifier - It explains the access kind of method and this is optional for use.
Parameter List
It is the list of parameters, that is the number, order, and type of parameters of the method. These are the optional methods that consist of zero parameters.
method body
An method body describes what a method performs with the statements.
nameOfMethod
It is the method name. A method signature includes the parameter list and the method name.
Method Calling
Generally, to use a method, it must be called. There are two types through which a method can be called, to be precise, a method that returns the value and a method that doesn't return anything.
The steps of method calling are simple.
In case assuming that the program calls a method, then the program controls are also relocated in the called method. Then, a called method sends the control to a caller on two conditions and they are:
It sends back the statement that is executed. It reaches to a method that uses the ending closing brace.
A method that returns the void is termed as the call to statement.
For Example
This method sends back the value that shall be understood using the below example
int result = sum (6,9)
A Void Keyword
A Void Keyword permits us to create methods that do not return the value. Here, in the example given below, let us take into consideration the void method for the methodRankPoints. It is a void method that can't refute the value. To call, the void method, it should be a statement, i.e it must be methodRankPoints (255.7); This is the Java Statement that ends with the semicolon as shown below
Passing the Parameters by Value
When you work under a calling process, the arguments should be passed. It should be in the structure that is similar to the corresponding parameters in the method of the specification. A parameter shall be passed utilizing the reference or value. While passing the Parameters, the value indicates calling the method with the parameter. This is how the argument value is passed to the parameter.
Method Overloading
In case if a class has more than two methods with the same name, but with different parameters, this is known as method overloading. This is a contrast to an overriding method. When using the overriding method, you should have the same method type, name, and the number of parameters.
By using the Command-Line Arguments
At times, you may need to pass a few information to the program where you run them and it is accomplished by passing the command-line arguments to main().
The Command-Line argument is the piece of information that directly pursues a program's name on a command line when it is executed. Also, it is easy to access the command-line arguments from the Java Program. It stacks the Strings in a String array that is passed to the main ()
The Finalize () Method
It describes the method that shall be invoked just before the object's final elimination by using a garbage collector. A method is called for finalize () and this can be utilized for checking that the object is eliminated neatly.
For instance,
You may use the finalize() for making sure that the open file is gained by an object that is closed. In case if you want to add the finalizer to a class, you must describe the finalize() method. The Java runtime calls the method while it is about to recover the object of that class. Inside a finalize() method, you can mention the actions that should be executed before the object that is going to be eliminated.
Over here the keyword is shielded with the specifier that halts the access to the finalize() by code that is described outside the class.
It denotes that you can determine when or how they finalize would be executed. For instance, by any chance, if your program ends prior to the process of garbage collection, then at any cost the finalize () can not be executed.
Java I/O
The Java I/O package comprises almost all the classes you may require to execute the input and out in Java. And all these streams interpret the input source and the output destination. The Java stream in the Java.io package backs the data like an object, localized characters, and primitives.
Stream
The Stream is the sequence of the Data. In Java, you can stream the composed of bytes.
In Java, there are 3 streams that are developed automatically and all these streams are hooked with a console.
- System.in - Standard Input Stream
- System.out - Standard Output Stream
- System.err - Standard error stream
The Code that is used for getting the Input from the Console is
int i=System.in.read();//returns ASCII code of 1st character
System.out.println((char)i);//will print the character
The Code for printing the error message and output to the consoles are
System.out.println("simple message");
System.err.println("error message");
The difference between the Input Stream and Output Stream
Input Stream
The Java Application basically uses the input stream for reading the data from the source, it can be anything like a socket, peripheral, an array, or a file.
Output Stream
The Java Application uses the Output Stream for writing the data for a specified destination, it can be anything like a socket, peripheral, an array, or a file.
The Diagram that is given below helps you to comprehend the Java Input and Output Stream easily
Input Stream Class
An InputStream Class is the abstract class and it is a superclass of all the classes that depicts the input stream of the bytes.
The Useful Approaches of the Input Stream
Method |
Description |
public abstract int read()throws IOException |
It reads the next byte of the data from an input stream. It rebounds -1 by the end of a file. |
public void close()throws IOException |
It is used for winding up the present Input Stream. |
public int available()throws IOException |
This sends back the estimate of a number byte and it can be used for reading it from the present input stream. |
Output Stream Class
It is an abstract class. This is the Superclass of the gross classes that interprets the output of the stream of bytes. The output stream acquires output bytes and returns back a few to them for syncing.
The Useful Approaches of the Output Stream
Methods |
Description |
public void write(int)throws IOException |
This is used for writing the byte for the present output stream. |
public void close()throws IOException |
It is used for winding up the present Output stream. |
public void write(byte[])throws IOException |
It is used for writing the Array of the byte to the present output stream. |
public void flush()throws IOException |
It crimsons the present output stream. |
Java Exceptions
Usually, the Exceptions are the problems that crop up during the time of an execution program. Suppose if an Exception takes place the normal flow of a program will be disrupted and that application terminates unnaturally, that is not advisable, hence these exceptions should be handled carefully.
The Exception can take place because of numerous reasons. Below is the situation where the exception takes place,
- In case if the user has entered into unauthorized data (data i.e invalid)
- If a Network Connection has been lost in between the communication or if the JVM is out of memory.
- A file that is needed to be opened but if you are unable to identify it.
The Exceptions here are developed because of the program error, user error, or other physical resources that failed in some manner.
Based on this situation, in this Java Programming Tutorial, we have categorized the Exception into three and you should comprehend them to know how these exception handling work in Java,
- Checked Exceptions
- Unchecked Exceptions
- Errors
Checked Exceptions
The Checked Exceptions is the exception that is verified by the compiler during the time of compilation and these are termed as the compile-time exceptions. One can not avoid these exceptions just like that, the programmer must take care of these exceptions.
Unchecked Exceptions
The Unchecked Exceptions are the exceptions that take place at the time of execution. It is termed as the Runtime Exceptions. It includes the programming bug-like errors and other irregular uses of the API's The Runtime Exceptions are neglected during the compilation.
Errors
These are not the kind of exceptions, but these are the problems that appear beyond the limit of a programmer or a user. The Errors are generally ignored in your code as you can hardly perform anything about the error. For instance, if the Stack Overflow takes place, the error would set in and also it shall be ignored during the time of compilation.
Exception Hierarchy
Every Exception class is the Subtypes of java. lang. Exception class. An Exception class is the hierarchy of a throwable class. Apart from an exception class, there are additionally subclasses that are termed as Error and it is borrowed from a Throwable class.
The Errors are the abnormal conditions that take place at times of severe failures and these can not be managed by Java programs. The Errors are navigated to denote the errors that are generated by the runtime environment. For instance, An, JVM is usually out of Memory. Usually, the programs may not be able to recover from the errors. An Exception class consists of two main subclasses and they are the RuntimeException Class and IOException class.
Now let us see a brief description of the Exception Methods
- public Throwable getCause() - It helps in sending back the cause of exceptions that are depicted by the Throwable Object.
- public void printStackTrace() - It helps in printing the result of the toString() with a stack trace of the error output stream or System.err.
- public String toString() - It refutes the name of a class that is concatenated to the result of the getMessage().
- public String getMessage() - This refutes the detailed text of the exception that has taken place. It is the text which is booted on a Throwable constructor.
- public Throwable fillInStackTrace() - It fills in the Stack of the Trace of this Throwable object with the present stack trace and it adds to the before the information on the stack trace.
- public StackTraceElement [] getStackTrace() - It refutes the arrays that comprise the element from a stack trace. An element on the index 0 epitomizes the top of a call stack. The ending element in an array epitomizes the type of the bottom call stack.
Catching Exception
This approach captures the Exception using the blend of catch and tries keywords. The Codes that are not beyond the try/catch block are termed as the protected code and the syntax for using a try/catch seems more or less similar.
A Code that is prone to an exception that is placed on the try block. Whenever the exception takes place, it is managed by the catch block that is related to it. All the Try blocks must be followed automatically using a catch or the final block.
The Catch Statement engages in asserting the kinds of exceptions that you are trying to capture. If the exception takes place on the protected code, then the catch block that supersedes a try is verified. If a type of exception has taken place in a catching book is listed, then the exceptions are passed for catching the block that is more like the argument than it is on to the passed method of a parameter.
Multiple Catch Blocks
The Try Block shall be superseded with different catch blocks.
Throw/ Throws keyword
If a method can not tackle the checked exception, then the method should term itself using the throw keyword. A throw keyword crop ups at the conclusion of the method signature.
Common Exceptions
In Java, you can describe two categories of errors and exceptions.
- JVM Exceptions - It is the Exceptions/ errors that are logically and exclusively thrown by a JVM. For instance: NullPointerException, Class Cast Exception, an ArrayIndexOutOfBoundsException.
- Programmatic Exception - These are the exceptions that are thrown directly by an application or by the API Programmers. Example: IllegalStateException, IllegalArgumentException.
Java Training in Coimbatore at FITA Academy provides extensive training of the coding and scripting practices of the Java code under the mentorship of expert Java professionals with certification.
Java Inner Classes
In this Java Tutorial, like the Variables, Methods of the class can also have the other class as an owned member. Writing the class in something else is permitted in Java. A Class that is written in is known as a nested class. The Class that occupies an inner class is termed as an outer class.
Syntax
Below is the Syntax for the inner demo and outer demo in the nested class
The Nested Classes are further classified into two types and they are
- Static Nested Classes
- Non-Static Nested Classes
- Static Nested Classes - It is the Static Member of a Class
- Non-Static Nested Classes - It is the Non-static Member of the Class
Static Nested Classes
The Static Inner Class is the Nested class that a Static Member of the Outer Class. This shall be accessed past without calling the outer class and by using the other static members. Unlike the Static Members, the static nested may not need to have access to the instance methods and variables of an outer class.
Syntax
Inner Classes ( Non-Static Nested Classes)
The Inner Classes are the Security systems in Java. It is a known fact that the class shall not be combined with an access modifier in private, however, when you accept a class in the process of another class, later the inner class shall be made as private. It is also used for ingressing the private members of the class. It is of three kinds based on the kinds and they are
- Inner Class
- Method-local Inner Class
- Anonymous Inner Class
Inner Class
Building an inner class is more simple. All you need is to write the class in a class. In contrast to a class, the inner class shall be private and when you declare the inner class is private, you can not access it from the object that is outside the class.
Accessing Private Members
As specified earlier, the inner classes are used for pervading the private members of the class. In case, the class consist of a private member for pervading them, then write the inner class inside it and then return them to the private members from the method in the inner class, for example, getValue() and certainly from the other class you can call a getValuw() method to the inner class.
Method-Local Inner Class
One of the prime features of Java is that you can write the Class in a method and it would mostly be of local kind. The Local variables and the scope of an inner class are curbed within a method.
The Method-Local inner class shall be called only with a method where an inner class is described.
Anonymous Inner Class
The Inner class that is stated without the class name is called Anonymous Inner Class. In the event of Anonymous Inner Class, it should be stated and called at the same time. Usually, it is used while you are required to nullify a method of the interface or class.
Java Inheritance
The Java Inheritance is the System on which the object obtains every behavior and properties of the parent object. This is the most important part of the OOPs.
The theory behind the inheritance in Java is you can build new classes that are built on the existing classes. If you derive from the existing class, then you can rephrase the fields and methods of a parent class. Further, you can also enumerate fields and methods in the current class as well.
Usually, the inheritance depicts the IS-A relationship that is comprehended as the parent-child relationship.
Reason to use the Inheritance in Java
- It is used for Method Overriding
- Code Reusability
Different Terms used in Inheritance
- Child Class/Sub Class - It is a class that derives from the other classes. This is also known as the derived class, child class, or the extended class.
- Class - The Class is the set of objects that have general properties. It is the blueprint or template from where the objects are built.
- Parent Class/ Super Class - It is the Class from where the subclass derives the features. This is also termed as the Parent Class or the Base class.
- Reusability - The very name itself indicates the structure of reusability and it allows you to reuse the methods and fields of an existing class where you can build the new class. Also, you can use similar methods that are already described in the earlier class.
The Extended keywords denote that you are building the new class that is inherited from the existing class. The term "extends" denotes an increase in functionality. The term Java is the class that is derived and it is called the superclass or parent, and this new class is termed as the subclass or child.
Java Overriding
In this Java Programming Tutorial let us see about the Overriding. In case if the class is derived from the method of its superclass, then you have the possibility to override a method that is given and that which is not marked as the final. The advantage of the overriding is the capacity to describe the behavior that is specific to a subclass type and it means the subclass shall deploy the parent class method on the basis of the needs. Based on the object-oriented terms, overriding here indicates overriding the performance of the existing method.
Rules should be followed for implementing the overriding method
- The Methods that are stated as final can not be overridden.
- An Argument list must be completely similar to that of an overridden method.
- An Instance method shall be overridden only when it is derived from the subclass.
- A return kind must be similar or the subtype of a return type should be stated on the original overridden method on the superclass.
- The Methods that are stated as the Static shall not be overridden however, it can be redeclared again.
- When the method is unable to be derived then it shall not be overridden.
- An access level shall not be restrictive and it can not be overridden for the method's access level. For instance: When the Superclass method is not stated publicly, then overriding the method on the subclass shall not be either protected or private.
- The Subclass is a completely different package that can override only a non-final method and it is stated as protected or public.
- The possibility of overriding a constructor is impossible.
Java Polymorphism
Polymorphism means the capacity of the object to hold any form. The general use of polymorphism takes place in OOPs where the parent class reference is used for indicating the child class object.
All the Java objects shall pass above one IS-A test that is termed to be polymorphic. Almost, every object in Java is Polymorphic since the object shall pass the IS-A test on its own kind and for a class object.
The most important factor is that it is possible only to access the object via a reference variable. And the reference variable is of only one type. If it is stated once, then the kind of reference variable shall not be modified.
A reference variable shall be assigned again to the other objects that are given is not stated as the final. The kind of reference variable shall find the methods through which it can invoke the object.
The reference variable shall indicate any object of the stated type or the subtype of the stated type. The reference variable shall be stated as the interface or class type.
For Example
public interface Vegetarian{}
public class Animal{}
public class Elephant extends Animal implements Vegetarian{}
Over here, the Elephant is termed to be polymorphic as it consists of different inheritance. The below is true for the above-given examples:
- A - Elephant IS - A Animal
- A - Elephant IS - A Vegetarian
- A - Elephant IS -A Elephant
- A - Elephant IS - A Object
If you apply the reference variable details to the Elephant Object reference, then the following declarations are considered to be legal
For Example
Elephant e= new Elephant();
Animal a = e;
Vegetarian v = e;
Object o = e;
All reference variables e, a, v, o, indicate the same Elephant object in the stack.
Java Abstraction
Abstract Class
The Class that is stated within the abstract keyword is called the Abstract class in Java. You have abstract and non-abstract methods. In this Java Tutorial, let us first get a clear understanding of what abstraction is all about.
Abstraction
It is the method of hiding the operation details and displaying only the functionality to a user.
It displays only the vital things to a user and shields the internal facts, like sending a message where you enter a text and send it. The users wouldn't be aware of the internal processing of a message delivery process. Abstraction permits you to concentrate on the performance of the object rather than the process that is involved in a performance.
Methods to reach Abstraction
There are two different methods for achieving abstraction in Java and they are
- Abstract Class (0 to 100%)
- Interface (100%)
The Abstract Class in Java
The Class that is stated as the abstract is called the abstract class. You can have abstract & non-abstract types. It should be extended and this method should be implemented. And it can't be called.
Java Notes for beginners
- The Abstract Class should be stated with the abstract keyword.
- It consists of Abstract & Non-abstract types.
- This shall not be called.
- An Abstract class could have static and constructor types as well.
- It has final methods that shall force a subclass for not changing the body of a method.
Rules of the Java Abstract Class
- The Abstract Class should be stated within the abstract keyword
- The Abstract Methods have final methods.
- The Abstract Class shall have the constructor and static methods
- The Abstract Class can have the Abstract and Non-Abstract types
- This can be called.
For Example of Abstract Class
abstract class A {}
Abstract Practice in Java
The Method that is stated as abstract and that which does not have the operation is called the abstract method.
For Example
abstract void printStatus();//no method body and abstract
Example
Over here, the Cycle is the abstract class that consists of only one abstract process run. The Implementation is given by the Lady Bird Class.
abstract class Cycle{
abstract void run();
}
class Lady Bird 4 extends Bike{
void run(){System.out.println("running safely");}
public static void main(String args[]){
Cycle obj = Lady Bird4();
obj.run();
}
}
Java Encapsulation
Encapsulation is one of the basics of the four OOP concepts. The rest are abstraction, polymorphism, and inheritance.
The Encapsulation in Java is the structure of wrapping data and code that are performing on the data together as a single unit. Over here the variables of the class are shielded from the other classes and it is possible to pervade via the methods of its present class. Hence, this is called Data hiding.
For achieving the Encapsulation in Java
- It should state the variables of the class as the private.
- It gives the Public Getter and Setter methods for viewing and modifying the values of variables.
Advantages of Encapsulation
- It Declares the variables of the class as the private
- It enables public getter and setter manners to view and modify the values of the variables.
Java Interfaces
The Interface is the reference kind in Java. It is identical to the class and the compilation of the abstract methods. The class shall instrument interface, and derive the abstract methods to an interface. Further with the Abstract methods, the interface consists of the static method, nested type, constants, and default methods. The Method bodies only prevail for the default and static methods.
Writing the interface that is identical to Class. The class defines the behavior and characters of an object. The interface comprises the attributes of the class instruments.
Except for the Class which instruments an interface is abstract, of all methods of an interface that has to be described in a class.
The Interface is similar to that of a class in the below methods:
- An Interface is usually written in the file that is within the .java extension of a similar interface that adheres to the name of a file.
- The Interface shall consist of more number of methods.
- Usually, the byte code of the interface surfaces in the .class file.
- Interfaces that surface in the packages and to its interrelated bytecode file should be found in the directory structure that adheres to the package name.
Yet the interface is so different from the class in multiple ways and it includes
- It is not possible to call the interface
- All the methods in the interface are abstract.
- An Interface will not comprise any instance fields. A field that appears on the interface must be stated both in Final and Static.
- The interface does not consist of any constructors.
- The interface shall expand the different interfaces.
- An interface is not only expanded by class, but it is instrumented by the class.
- The Interface can extend different interfaces.
Declaring an Interface
The interface keyword is used for declaring the interface
Implementing Interfaces
When the class instruments the interface, you can use the class as the signing contract and adhering to perform a particular behavior of an interface. In case, when the class fails to execute all functions of an interface, then the class should mention itself as abstract. The Class utilizes the implement keyword for instrumenting the interface. An implement keyword surfaces in a class declaration and it expands the portion of a declaration.
In case the overriding methods are described in the interface, then numerous rules have to be followed
- An implementation Class itself must be abstract and so the interface type may not be required to implement.
- A signature of an interface method and similar return type or a subtype must be maintained while overriding methods.
- A checked exception may not be declared on the implementation methods apart from the ones which are declared by a subclass or interface method of the ones that are stated by an interface method.
In case if the implementation interfaces, then there are numerous rules
- A class can instrument above one interface at a time.
- A class shall extend can broaden only one class and it implements more than one interface.
- The Interfaces shall extend the other interface in the same way as that of the class would extend the other class.
Extending Interfaces
The Interface shall extend other interfaces that are similar to the way that the class shall extend to the other class. An extended keyword is utilized for expanding the interface and a child interface that derives the method of a parent interface.
Extending Different Interfaces
The Java Class shall broaden only one of the parent classes. The different inheritances are not permitted. The Interfaces are no class, and the interface shall extend above one parent interface.
An Extend keyword is utilized only once and a parent interface is stated in the comma-separated list.
Tagging Interfaces
The general use of an extending interface takes place when a parent interface does not consist of any methods.
Java Training in Gurgaon at FITA Academy upskills your knowledge of Java Coding and its structure extensively under the training of real-time Java Professionals with certification.
Packages
The Packages are utilized in Java to avoid the conflicts in access and control for making the location/ searching and the use of the classes, annotation, enumerations, and interfaces.
The Package shall also be defined as the set of related types like (enumerations, classes, interfaces, and annotations ) that give access to the namespace and protection management.
Other existing packages that are comprised in Java are
- java.io - The Classes for the Output and Input functions are wrapped in the packages
- java-lang - These packages are the fundamental classes
The Programmers are defined on the package of bundle groups such as interfaces/ classes, etc.
This is a good method of practice that is associated with classes that deploy a programmer and you can identify the interfaces, annotation, enumerations, and classes.
As the package develops the new namespace there will not be any conflicts in names and also the names that are in other packages. Utilizing these packages is simpler to provide entry control and it is easy to find the other associated classes.
Creating Packages
When you create a package, you must select the name of a package and consists of the package statement with a name on the top of all the source file that consists of the enumerations, interfaces, classes, and annotation types you are planning to add into a package.
A package statement should be the starting line of the source file. There can also be only a single package statement on every source file and this is applicable for all kinds of files.
Supposedly if the package statement is not utilized then the interfaces, annotation, class, and enumeration types would not be placed on a present default package.
For compiling the Java programs along with the package statements you can use the -d option as given below
javac -d Destination_folder file_name.java
Then the folder with the provided package the name is developed on the definite destination and this is gathered on the class files that and would be placed on the folder.
The Import keyword
If the class is required you must utilize the other class on a similar package, the package may not be used. The Classes on a similar package can identify the other without any exclusive syntax.
Structure of the Directory Package
The two important results that take place in the class of the package
- The name of a package that turns out to be part of the name of a class and should be mentioned in the earlier section.
- The name of a package should adhere to the directory structure and it should cohere with the bytecodes that reside along with it.
Below is the simple way to handle your files in the Java
Place the Source code to an interface, enumeration, or the annotation kind on the text file that the name is simpler than the name of a kind and on the extension is.java.
For Instance,
// File Name : Apple.java
package fruit;
public class Apple {
// Class implementation.
}
Output
....\fruit\Apple.java
The qualified class and the pathname shall be as follow:
- Class name -> fruit. Apple
- Pathname -> fruit\Apple.Java ( in the windows system)
Usually, a company uses the reverse Internet domain name for the package names.
For Example
If a company has the Internet domain name - windows.com, then all the package names shall begin with the com. windows. All the components of a package name must adhere to the subdirectory.
Advanced Java Tutorial
Data Structures
The Data Structures that are given by the Java utility package are robust and can execute a range of functions. The Data structures comprise of the below classes and interface,
- Vector
- Stack
- Enumeration
- BitSet
- Hashtable
- Properties
- Dictionary
Vector
A Vector class has more resemblance to the traditional Java array, apart from that it can also manipulate as needed, and it can consist of new elements. Just like an array, the elements of the vector object are controlled through the index in a vector. The best part of using a vector class is you need not care about setting it to the particular size on creation, it can adjust (shrink/enlarge) itself when it is required.
Stack
A Stack Class deploys the LIFO method i.e Last-in-first-out, a stack of elements. Over here the Stack is the vertical stack object, where you can pass the new elements and get them stacked on the above of others. In case if you pull the element off a stack, then this moves to the top. To make it easier, the closing element that you add to a stack is the one that appears like the back off.
Enumeration
It is not a data structure by itself, but enumeration is more important within the conditions of the other data structures. An Enumeration is an interface that describes the means for recovering the successive elements from the data structure.
For Example, Enumeration describes the method that is termed as the nextElement and it is used for obtaining the immediate (next) element in the data structure queue that consists of different elements.
BitSet
A Bitset Class deploys the set of flags or the bits that could be fixed and cleared individually. It is more useful in case you need to keep up to the set of Boolean values. You can easily designate the bit all the value and it can be fixed or cleared as appropriate.
Hashtable
A Hashtable Class gives the space for organizing the data on the basis of the user-defined key structure.
For instance, on your address list hash table, you can sort, save the data based on the key like ZIP code instead of the particular person's name. The exact meaning of the keys in respect of the hash table is completely on the application of a hash table and the data it consists of.
Properties
The Properties are the subclass of the Hashtable. It is used for handling the list of values on which a key is a string and the string is also the value. Apart from this, the properties class is used by other Java Classes.
Dictionary
A Dictionary class is the abstract class that describes the data structures to map the keys to the corresponding values. It is more useful in a situation where you are unable to get access to the data through a specific key instead of the integer index. As the Dictionary Class is abstract, it gives a framework that is key-mapped data structure instead of the particular implementation.
Java Collections
A Collection in Java is the framework that gives the architecture for the store and it modifies the objects in the groups. The Java Collection shall accomplish all the operations that you must perform on the data like insertion, sorting, searching, deletion, and manipulation.
The Collection of the framework was curated to match the different goals like
A Framework permits numerous types of collection for working in an identical manner and it has a high degree of interoperability.
A Framework has to perform extremely well. The Implementation of the basic collections like ( linked lists, trees, dynamic arrays, and hashtables) must be highly efficient.
A Framework was created to extend or adapt to the collection in an easier manner.
Finally, the complete collection of a framework is curated on a set of common interfaces. There are numerous standard implementations like TreeSet, LinkedList, and HashSet, and these interfaces are given so that you can use them as they are and also deploy them on your collection when you choose.
The Collection of Framework is the unified architecture that is used for manipulating and interpreting the collections. All the Collections of the framework comprises of the following,
- Algorithms - It is the common method that is used for performing computations like sorting or searching an object that shall instrument the collection interfaces.
- Interface - It is the abstract data type that interprets the collections. The Interfaces permit the collections to modify freely irrespective of the facts of the representation. It is a common thing that in the Object-oriented language, an interface would build the hierarchy.
- Implementations - It is the detailed implementation of the collected interface and it is the renewable data structures.
Further, a framework describes different map classes and interfaces. Although the maps are not collections that are in appropriate use of a term, however, it is completely combined with collections.
The Collection Interface
In this Java Programming Tutorial let us see the collections framework that describes numerous interfaces.
- Map - It aids in mapping the distinct keys to the values.
- Collection Interface - It allows you to work with a set of objects and it is on the top list of the collection hierarchy.
- Set - It extends the Collection for handling sets that contain a distinct element.
- SortedSet - It helps in extending the Set for managing the sorted sets.
- Enumeration - It is the legacy interface that describes the method through which you shall enumerate elements from the collection of objects. The bequest interface is antiquated through Iterator.
- Sorted Map - It helps in enlarging the Map for managing the keys in ascending order.
The Collection Classes
Java supports you with you a set of benchmark collection classes that instrument the Collection interfaces. The Classes gives complete implementation that is used as it is and another abstract class, that gives the skeletal implementations which are used as the beginning points to create the definite collections.
Some of the Standard Collections are mentioned below
- Abstract List - It aids in extending the AbstractCollection and it deploys more List Interfaces.
- Abstract Collection - It deploys a more Collection interface.
- Abstract Sequential list - This Extends the AbstractList for the use by the collection that applies sequence instead of the random access of elements.
- Array List - It instruments the influential array on broadening the AbstractList.
- Linked List - It instruments the linked list on expanding the AbstractSequentialList.
- HashSet - It Extends the AbstractSet to make use of the hash table.
- TreeSet - This deploys the Set that is stored in the tree. It extends the AbstractSet.
- Linked HashSet - It lengthens the HashSet and permits the insertion-order iterations.
- Abstract Map - It deploys maximum Map Interface.
- Tree Map - It Expands the Abstract Map for utilizing the tree.
- Hash Map - This broadens the AbstractMap to utilize the Hash table.
- Weak Map - It expands the AbstractMap for utilizing the hash table that is in the limit of weak keys.
- IdentityHashMap - This broadens the AbstractMap and it makes use of the reference equality while compared to the documents.
- LinkedHashMap - This Expands the Hashmap for permitting the insertion-order iterations.
Below are the classes that give the skeletal implementation of the core collection interface for reducing the effort that is needed to deploy them,
- AbstractSet
- AbstractMap
- AbstractCollection
- AbstractList
- AbstractSequentialList
Collection Algorithm
A Collection Framework explains various algorithms that are practiced on the maps and collections. It is characterized as Static methods in the limits of the collection class.
The various methods that shall throw the ClassCast Exception that takes place when the experiment is made for analyzing the incompatible types or the UnsupportedOperationException, that takes place while the attempt is made for changing the unmodifiable collections.
Iterator
Usually, you may need to wheel to the elements in the collections. For instance, you may be required to demonstrate all elements. The simplest way for performing this is that you can deploy the iterator, which is actually the object that instruments either the Listiterator interface or the Iterator
An Iterator permits you to go through the collection or remove elements. The ListIterator shall broaden the Iterator for allowing modification of the elements and the traversal of the list.
Comparator
Both the TreeMap and the TreeSet stack the elements in a sorted manner. The Comparator demonstrates accurately everything about sorted order. It basically helps you to sort for the provided collection by any number or through multiple ways. Furthermore, this interface shall be applied for sorting any instance of whatever class.
Java Networking
It is the notion of bridging two or above computing devices in sync so that you can share the resources. The Java Socket Programming gives the competency to share data between various computing devices. The two major benefits of Java Networking are,
- You share the resources easily.
- You have the Centralized Software Management kit.
Java Networking Technology
In this Java Tutorial let us see the broadly-used Java Networking Terminologies
- Port Number
- MAC Address
- Protocol
- IP Address
- Socket
- Connection-oriented protocol
Port Number
The Port number is utilized distinctly for determining various applications. It operates as the endpoints of communication between applications. A port number is related to the IP address to communicate between two applications.
MAC Address
The MAC address ( Media Access Control) is the distinct identifier of the Network Interface Controller. The Network Node shall have a different NIC however everything with the distinct MAC.
Protocol
The Protocol is the compilation of rules that are generally used in communication. For instance - FTP, Telnet, TCP, SMTP, and POP.
IP Address
The IP Address is the distinct number that is designated to the node of the network. For example - 192.168.0.1 and this is compiled of the octets that length between 0 to 255. This is the logical address that could not be changed.
Socket
The Socket is the endpoint between the two ways of communication.
Connection-oriented protocol
On the Connection-oriented protocol, the affirmation is sent by a receiver. Even though it is slow, this is reliable. For Example - TCP.
Connectionless protocol
In the Connectionless protocol, the acknowledgment will not be sent by a receiver and it is not reliable but this is faster. For Example - UDP.
Java Socket Programming
The Java Socket Programming is utilized for communication between the applications which run on different JRE.
The Java Socket Programming can either be one that is connectionless or connection-oriented. The ServerSocket Classes and Socket is used for connecting the oriented socket programming. For connection-less socket programming, you can use the DatagramPacket and DatagramSocket.
A Client in Socket Programming should know two pieces of information and they are
- Port Number
- IP Address of the Server
Over here, we have demonstrated the example for one-way server and client communication. And here the Client dispatches the message to a server, and the server reads the text and later prints it. Exactly, here two classes are utilized and they are ServerSocket and Socket. A Socket Class is used for communicating to the Server and Client. With this, you can write and read the message. A ServerSocket Class is utilized at the server-side. An Accept() method of a ServerSocket Class halts the console till the client is connected. Later on the successful connection to the client, it sends the instance of the Socket to the server-side.
Socket Class
The Socket is the endpoint of communications among machines. A Socket Class is used for building the socket.
Some of the important methods
Methods |
Description |
public synchronized void close() |
It shuts down the socket |
public InputStream getInputStream() |
This sends the InputStream that is affixed along with the socket. |
public OutputStream getOutputStream() |
It sends back the Output stream that is attached to the socket. |
Server Socket Class
A ServerSocket Class is utilized for building the server socket. It is the object that is used in building the communication along with the clients.
Some of the important Methods are
Methods |
Description |
public Socket accepts () |
It refutes the socket and it builds the connection among the client and server. |
public synchronized void close() |
It shutdown the Server socket. |
Java URL
The abbreviation of the URL is Uniform Resource Locator. This directs to the resource that is on the World Wide Web. For instance,
https://www.fita.in/java-training/An URL Consists of numerous information and they are
- Port Number - It is the optional character. If you write https://www.fita.com.70/trainingcenter/ the number 70 is the port number. In case there is no port number that is mentioned on the URL then it returns to -1.
- Directory Name or File Name - Here the index. jsp is the name of the file
- Protocol - The HTTP is protocol.
- IP Address or Server name - www.fita.in is the name of the server.
Predominantly used Java URL Class
Method |
Description |
public String getFile() |
This sends back the file name of the URL. |
public String getProtocol() |
It refutes the protocol of an URL. |
public String getPort() |
This sends back the Port Number of the URL. |
public String getHost() |
It refutes the hostname of the URL. |
public String toString() |
It sends back the String interpretation of the URL. |
public String getAuthority() |
It sends back the authority of This sends back the content of anURL. |
public String getDefaultPort() |
This rebound the query string of URL |
public String getQuery() |
It refutes the query string of an URL. |
public Object getContent() |
This sends back the content of an URL. |
public boolean equals(Object obj) |
It compares the URL with a given object. |
public URI to URI() |
It sends back the URI of an URL. |
public String getRef() |
This sends back the reference or the anchor of an URL. |
Java InetAddress Class
The Java InetAddress Class demonstrates the IP address. A java.net.InetAddress also gives the methods for getting the IP of any hostname. An IP address is illustrated by the 32-bit or by the 128-bit which is the unsigned number. The instance of the InetAddress depicts the IP address that correlates with the hostname. There are two kinds of address types and they are Unicast and Multicast. A Unicast is the qualifier of the single interface where a Multicast is the qualifier of the set of interfaces. Further, the InetAddress consists of the cache mechanism that aids in storing the successive and non-successive host-name resolutions.
Important Methods of the InetAddress Class
Method |
Description |
public String getHostName() |
This sends back the hostname of an IP address. |
public String getHostAddress() |
This helps in refuting the IP address in the String format. |
|
This helps in sending back the instance of the InetAddress that consists of the local address and hostname. |
Java - Sending Email
For sending the e-mail you should use the Java Application that is close enough, to begin with, where you must have installed the Java Activation Framework or the Javamail API to your machines. For downloading the recent version you can use Java's standard Website.
On downloading it successfully, you can unzip those files in a newly developed top-level directory where you can find out the total number of the jar files for both applications. All you have to do is that you must add the activation.jar and mail.jar to your CLASSPATH.
Java Multithreading
Java is considered to be one of the multi-threaded programming languages that means you can build a multi-threaded program using Java. The Multi-threaded program consists of two or more parts that shall run simultaneously and all the parts shall manage the various tasks simultaneously by making optimal use of the resources that are provided and when the computer has numerous CPUs.
The word multitasking means where there are numerous processes that share common operations of all resources like CPU. The Multi-threading widens the concept of multitasking into applications where you shall partition few applications in the limit of the single application and into individual threads. All the threads are capable of running collar OS aids in partitioning the operating period in the applications as well as to all the threads that are within an application.
Generally, the multi-threading allows you to write in a manner where different activities shall proceed with the same program altogether.
The Wheel of the Thread
Usually, a thread undergoes different stages. The flow-chart that is given below precisely explains the lifecycle of the thread.
Below are the detailed description of the Thread Lifecycle
- New - The New Thread take starts its life cycle with the new states. It prevails in this state up to when a program takes off with the thread and it is called a born thread.
- Runnable - Once the newly born thread begins its function, then the thread shall become runnable. The thread in this state is termed to be runnable as it is executing the tasks.
- Waiting - At times, the thread flux back to the runnable state when the other thread signals to the waiting thread to pursue the execution function.
- Time Waiting - The Runnable threads shall barge into the restrained waiting state for a definite period. The thread which is in this state shall flux back to a runnable state in case the allotted time interval is expired or while an event is awaiting its occurrence.
- Terminated - The Runnable thread moves into the terminated state where it tries to replete the tasks, if not then it would terminate itself.
Thread Priorities
All the threads in Java have a unique priority that supports operating the systems to identify the sequence through which the threads are being scheduled. The Java thread priorities are within the range of MIN_PRIORITY and MAX_PRIORITY. Where the MIN_PRIORITY is the constant of 1, and the MAX_ PRIORITY is the constant of 10. By default, all the threads are provided priority i.e NORM_PRIORITY which are constant of 5. The threads that are with higher priority are more important to the program and this should be designated to the processor time before the lower-priority threads. Yet, these threads' priorities shall not be guaranteed in the sequence on which the threads are executed; it depends more on the platforms.
Now in this Java Developer Tutorial, let us see how to build a thread on
In case, when your class is aimed for execution then the thread shall attain this implementation by the Runnable interface. Below are the fundamental steps in creating the thread,
Step-1
The first step you must do is that you should implement the run() method by enabling the Running interface. The method that gives the entry point to a thread and you shall upload the entire business logic to this method.
Syntax for run() method
public void run( )
Step-2
The second type will instantiate the Thread object by using the below constructor-
Thread(Runnable threadObj, String threadName);
The thread by is the instance of the class that deploys the thread name and the Runnable interface is the name that is provided to a new thread.
Step-3
If the Thread Object is developed then you can begin it by calling the start() method, which shall execute the call to the run() method.
Syntax for the start() method
void start();
Build the Thread by the Extending Thread Class
Java Applet Basics
The Applet is the Java Program and it runs on the Web browser. The Applet is the complete Java functional application as it consists of the entire Java API at its scraping.
Below are the major difference between the applet and the standalone Java application
- The Applet is the Java Class that boosts java. applet.Applet class.
- The Applets are devised to embed with the HTML Page.
- The main () method does not request the applet and the applet class may not define main ().
- If the user views the HTML page it consists of the applet and the code of the applet is installed into the respective user's machine.
- The JVM is needed for viewing the applet. The JVM shall either be a separate runtime environment or a plug-in to the Web browser.
- The Applets have stringent rules that shall be reinforced by a Web Browser.
- The Security of the applets is often attributed to the sandbox security when compared to the applet of the child playing on the sandbox with different rules which should be implemented.
- The JVM of the User's Machines develops the instance of an applet class and this requests different methods at the time of the applet's cycle.
- The Other Classes which the applet requires are downloaded into the single (JAR) Java Archive file.
The Applet Cycle
The Four methods in Applet Class provides you a framework in which you can build the serious applet:
- Start - It is the method that immediately calls the browser calls to init method.
- Init - It is the type that is aimed for initialization that is required for the applet. This is called later the param tags that are inside an applet tag that you have processed.
- Stop - It is the type that is immediately called when a user moves away from the page in which an applet sits. This can be called frequently on the same applet.
- Paint - It is requested automatically later by the start() method, and at any time the applet requires to refurbish itself in a browser. A paint() method is generally obtained from java.awt.
- Destroy - It is the method that is called if the browser closes down usually. When the applets are meant to be on the HTML page, then you can not usually leave the resources breach after the user leaves a page that consists of the applet.
The Applet Class
All Applet is the extension of java. Applet class. The base Applet class gives methods that are obtained from the Applet Class and it shall be called for obtaining the services and information from a browser context.
It consists of the following methods:
- Fetching the image
- Fetching the audio clip
- Plays the audio clip
- Resizing of the applet
- Getting the applet parameters
- Getting a Network location of an HTML file that consists of the applet
- Getting the Network location of an applet class directory
- It prints out the status text in the browser
- Initializes an applet
- Destroys the applet
- Request the information regarding the version, copyright, and author of an applet
- It requests the description of a parameter and the applet identifies it
- Ceases the execution of an applet
- Begins the execution of an applet
Further, the Applet Class shall give the interface on which a viewer or the browser gains the information of the applet and the control operation of the applet's execution.
An Applet Class that gives the default application of all the methods. Those applications shall be overridden when required.
Java Documentation
The Javadoc is the tool that comes with a JDK and this is used for developing Java Code documentation in the HTML format from the Java Source code and it needs the documentation of the predefined format.
The Java supports the following three types of comments.
- //'text - A compiler avoids everything from// till the end of a line.
- /*text*/ - A compiler avoids everything from the /* to*/
Java Useful Resources
Java Interview Questions
The Java Interview Questions and Answers are curated with a special focus to aid both the students and the working professionals who are preparing themselves for different Certification Exams and Job Interviews. This session is a complete compilation of the Important Java Interview Questions and Answers that are asked to fresher and experienced candidates in the interview.
Enlist the different access specifiers in Java?
- Public
- Private
- Default
- Protected
List the Advantages of Package in Java?
- The Packages evades the name clashes.
- It is easier to locate the related classes.
- The Packages give easier access control.
- You can also have the hidden classes which are not found outside the used package.
Name the types of constructors that are used in Java?
Java has two types of Constructors and they are,
Default Constructor?
Parameterized Constructor
Is it possible to make a Constructor final?
It is impossible to make the Constructor final.
Are the Constructors inherited?
No, the Constructors are not inherited.
For more: Click the link given below
Java Interview Questions and Answers.Java - Quick Guide
- The Java Tutorial - It is the practical set of guides to the programmers who need to use the Java Programming language for creating the applications.
- Free Java Download - Download Java to your desktop from the Computer now.
- SunDeveloper Network- This is the official website that is used for listing down the API documentation, books, latest Java technologies, and other resources.
- JavaTM 2 SDK - This is the Official Site for the JavaTM 2 SDK, the Standard Edition.
- Java Tutorial - It is the practical guide for the programmers who need to use the Java Programming language for creating the applications.
Interview Questions
Interview Questions on Digital Marketing Java Interview Questions For Experienced Selenium Interview Questions and Answers For Experienced Hadoop Interview Questions and Answers For Experienced Python Interview Questions and Answers For AWS Interview Questions and Answers For Experienced DevOps Interview Questions and Answers Oracle Interview Questions PHP Interview Questions For Freshers AngularJs Interview Questions RPA Interview Questions Software Testing Interview Question and Answer Mobile Testing Interview Questions and Answers Salesforce Interview Questions Networking Interview Questions and Answers Pega Interview Questions NodeJS Interview Questions Ethical Hacking Interview Questions Data Science Interview Questions and Answers Javascript Interview Questions Blue Prism Interview Questions SEO Interview Questions Android Interview Questions MS Excel Interview Questions Cloud Computing Interview Questions Cyber Security Interview Questions Interview Question on Tableau Power BI Interview Questions and Answers Artificial Intelligence Interview Questions and Answers Azure Interview Questions and Answers SQL Interview Questions and Answers Full Stack Interview Questions and Answers Dot Net Interview QuestionsFull Stack Interview Questions and Answers UI UX Interview Questions and Answers ReactJs Interview Questions Full Stack Interview Questions and Answers Linux Interview Questions
FITA Academy Branches
Chennai
Contact Us
- Chennai:93450 45466
- Bangalore:93450 45466
- Coimbatore:95978 88270
- Pondicherry:93635 21112
- Madurai:97900 94102
- Online:93450 45466
For Business
Testimonials
Resources
Follow Us
TRENDING COURSES
JAVA Training In Chennai Core Java Training in Chennai Software Testing Training In Chennai Selenium Training In Chennai Python Training in Chennai Data Science Course In Chennai C / C++ Training In Chennai PHP Training In Chennai AngularJS Training in Chennai Dot Net Training In Chennai DevOps Training In Chennai German Classes In Chennai Spring Training in ChennaiStruts Training in Chennai Web Designing Course In Chennai Android Training In Chennai AWS Training in Chennai
iOS Training In Chennai SEO Training In Chennai Oracle Training In Chennai RPA Training In Chennai Cloud Computing Training In Chennai Big Data Hadoop Training In Chennai Digital Marketing Course In Chennai UNIX Training In Chennai Placement Training In Chennai Artificial Intelligence Course in ChennaiJavascript Training in ChennaiHibernate Training in ChennaiHTML5 Training in ChennaiPhotoshop Classes in ChennaiMobile Testing Training in ChennaiQTP Training in ChennaiLoadRunner Training in ChennaiDrupal Training in ChennaiManual Testing Training in ChennaiWordPress Training in ChennaiSAS Training in ChennaiClinical SAS Training in ChennaiBlue Prism Training in ChennaiMachine Learning course in ChennaiMicrosoft Azure Training in ChennaiSelenium with Python Training in ChennaiUiPath Training in ChennaiMicrosoft Dynamics CRM Training in ChennaiUI UX Design course in ChennaiSalesforce Training in ChennaiVMware Training in ChennaiR Training in ChennaiAutomation Anywhere Training in ChennaiTally course in ChennaiReactJS Training in ChennaiCCNA course in ChennaiEthical Hacking course in ChennaiGST Training in ChennaiIELTS Coaching in ChennaiSpoken English Classes in ChennaiSpanish Classes in ChennaiJapanese Classes in ChennaiTOEFL Coaching in ChennaiFrench Classes in ChennaiInformatica Training in ChennaiInformatica MDM Training in ChennaiData Analytics Courses in ChennaiFull Stack Developer Course in ChennaiHadoop Admin Training in ChennaiBlockchain Training in ChennaiIonic Training in ChennaiIoT Training in ChennaiXamarin Training In ChennaiNode JS Training In ChennaiContent Writing Course in ChennaiAdvanced Excel Training In ChennaiCorporate Training in ChennaiEmbedded Training In ChennaiLinux Training In ChennaiOracle DBA Training In ChennaiPEGA Training In ChennaiPrimavera Training In ChennaiTableau Training In ChennaiSpark Training In ChennaiGraphic Design Courses in ChennaiAppium Training In ChennaiSoft Skills Training In ChennaiJMeter Training In ChennaiPower BI Training In ChennaiSocial Media Marketing Courses In ChennaiTalend Training in ChennaiHR Courses in ChennaiGoogle Cloud Training in ChennaiSQL Training In Chennai CCNP Training in Chennai PMP Training in Chennai OET Coaching Centre in Chennai
Are You Located in Any of these Areas
Adyar, Adambakkam, Anna Salai, Ambattur, Ashok Nagar, Aminjikarai, Anna Nagar, Besant Nagar, Chromepet, Choolaimedu, Guindy, Egmore, K.K. Nagar, Kodambakkam, Koyambedu, Ekkattuthangal, Kilpauk, Meenambakkam, Medavakkam, Nandanam, Nungambakkam, Madipakkam, Teynampet, Nanganallur, Navalur, Mylapore, Pallavaram, Purasaiwakkam, OMR, Porur, Pallikaranai, Poonamallee, Perambur, Saidapet, Siruseri, St.Thomas Mount, Perungudi, T.Nagar, Sholinganallur, Triplicane, Thoraipakkam, Tambaram, Vadapalani, Valasaravakkam, Villivakkam, Thiruvanmiyur, West Mambalam, Velachery and Virugambakkam.
FITA Velachery or T Nagar or Thoraipakkam OMR or Anna Nagar or Tambaram branch is just few kilometre away from your location. If you need the best training in Chennai, driving a couple of extra kilometres is worth it!
© 2020 FITA. All rights Reserved.