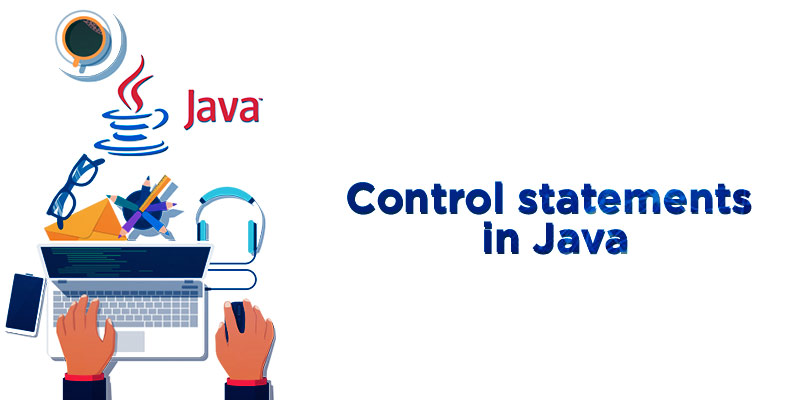
In this blog, I will explain control statements in java, when and how to use them with example programs.
Let us first understand what are control statements in java, and their categories
What are Control Statements in Java
As statements give instructions to the program, control statements constraints the flow of the program. Control statements are categorized as
If you understand what control statements are in java let us now understand each of these statements with example programs starting with Decision Making Statements In Java.
Decision Making Statements In Java
We have 4 decision making statements in java to control the flow of execution in Java as if, else, nested if else, and switch statement.
If statement in Java
This statement will execute the following block of code if the given condition is satisfied else it skips the if block of code.
Syntax:
if (condition)
{
statement; // will be executed if the condition is true, else skips to next statement
}
statement; // will be executed irrespective of the condition
Example Program for if statement
class IfExp
{
public static void main(String args[])
{
int x = 4;
if (x > 10)
System.out.println("If condition is true");
System.out.println("After if block");
}
}
// outputs: After if block
Let us hop to the next decision statement, if-else in java.
If-else statement in Java
Adding an else block in addition to if block will execute the else block only if the if block condition is not true.
Syntax
if (condition a) {
Statement a; //executed when condition a is true
}
else {
Statement b; //executed when condition a is false
}
Example program for the if-else statement
class IfElseExp {
public static void main(String args[]) {
int a = 19;
if (a > 100)
System.out.println("a is bigger than 100");
else
System.out.println("a is less than 100");
System.out.println("After if else statements");
}
}
Output for the above program
a is less than 100
After if else statements
Check out this Complete Java Course Online by FITA. FITA provides a complete Java course including core java and advanced java J2EE, and SOA training, where you will be building real time applications using Servlets, Hibernate Framework, and Spring with Aspect Oriented Programming (AOP) architecture, Struts through JDBC bundled with, placement support, and certification at an affordable price with an active placement cell, to make you an industry required certified java developer.
Let us hop to the next decision statement, nested if statements in java.
Nested if statements in java
The nested if statement will be executed if the condition is true and the parent if the condition is true.
Syntax
if (condition a) {
Statement a; //executed when condition a is true
if (condition b) {
Statement b; //executed when condition b is true
}
else {
Statement c; //executed when condition b is false
}
}
Example program for the if-else statements in java
class IfElseExp {
public static void main(String args[]) {
int a = 55;
if (a > 13) {
if (a % 2 == 0)
System.out.println("a is bigger than 13 and is even");
else
System.out.println("a is odd and bigger than 13");
} else {
System.out.println("a is less than 13");
}
System.out.println("After nested statements");
}
}
Output for the above program
a is odd and bigger than 13
After nested statements
Let us hop to the next decision statement, nested if-else statements in java.
Nested if-else statements in java
The nested if-else statements will be required when you need to test for more than 2 conditions.
Syntax
if (condition a) {
Statement a; //will be executed if condition a is true
}
else if (condition b) {
Statement b; //will be executed if condition b is true
}
.
.
else {
Statement c; //executed when none of the conditions is true
}
Example program for the nested if-else statements in java
class NEstIfElseExp{
public static void main(String args[]) {
int a = 23;
if (a > 23) {
System.out.println("a is bigger than 23");
} else if (a < 23) {
System.out.println("a is lesser than 23");
} else {
System.out.println("a is 23");
}
System.out.println("After if else if blocks");
}
}
Output for the above program
a is 23
After if else if blocks
These were the Decision making statements in Java, let us now jump to the Switch Statements in Java.
The Switch statement in Java
You can rewrite and define those nested if-else statements more clearly with a switch statement. It starts with an expression passed to switch and checks for various values with the case. Multiple cases should not have similar values nor should they be of different data types as of expression. A break statement can be used after any case, to cease the execution of the next cases.
A default statement can be passed to switch at the end to provide a default value if none of the cases match.
The default and break statements are optional here.
Syntax
switch (expression)
{
case value a:
Statement a;
break;
case value b:
Statement b;
break;
.
.
case value n:
Statement n;
break;
default:
Default statement;
}
Example program to implement a switch in java.
// Java program to illustrate switch-case
class SwitchExp {
public static void main(String args[]) {
int a = 7;
switch (a) {
case 7:
System.out.println("a is seven.");
break;
case 2:
System.out.println("a is two.");
break;
case 3:
System.out.println("a is three.");
break;
case 4:
System.out.println("a is four.");
break;
case 1:
System.out.println("a is one.");
break;
default:
System.out.println("a is greater than 4.");
}
}
}
Output for the above program
a is seven.
This was the Switch statement in Java, for making multiple decisions easier, let us now jump to the Looping Statements in Java.
Looping statements In Java
Looping is the method of performing a certain task repeatedly until the given condition is satisfied. There are three types of loops in java, while, do while, for loop.
while loop in Java
The compiler will execute the while block until the passed condition is true, or if the condition of the while loop is false it will never be executed. A boolean condition should be passed to the while loop.
Syntax
while (condition)
{
Statement;
}
Example program to implement the while loop in java.
class WhileExp{
public static void main(String args[]) {
int i = 1;
while (i <= 23) {
System.out.println(i);
i = i + 3;
}
}
}
Output for the above program
1
4
7
10
13
16
19
22
Let us hop to the next looping statement,do while statement in java.
do while loop
This is similar to while loop except that the condition of the while loop condition will be checked only after the block of code is executed at least once.
Syntax
do{
statement;
}while(condition);
Example program to implement the do-while loop
class Main {
public static void main(String args[]) {
int a = 17;
do {
System.out.println(a);
a = a + 2;
} while (a <= 17);
}
}
Output for the above program
17
Let us hop to the next looping statement,for loop statement in java.
for loop in java
for loops are used to repeat a certain task specified number of times along with a condition.
It consists of an initialization value, a condition, and increment or decrement of value.
Syntax
for (initialization; condition; increment/decrement)
{
statement;
}
Example program to implement for loop in java
class Main {
public static void main(String args[]) {
for (int a = 3; a <= 15; a+=3)
System.out.println(a);
}
}
Output for the above program
3
6
9
12
15
Let us hop to the next looping statement,for-each loop statement in java.
for-each loop in java
This is used to traverse or loop through the elements of the array, without the use of increment or decrement.
Example program to implement for-each in java
class Main {
public static void main(String args[]) {
int arr[] = { 1, 1, 2, 3, 5, 8, 11 };
for (int v : arr) {
System.out.println(v);
}
}
}
Output for the above program
1
1
2
3
5
8
11
These were the Looping statements in Java, for making iteration easier, let us now jump to the Branching Statements in Java.
Branching Statements In Java
Branching statements are used to make a jump from the normal flow of the program.
The Break statement in java
This statement is used to terminate the execution of looping statements after the break keyword.
Example program to implement the break-in java
class BreakExp{
public static void main(String args[]) {
int b = 0;
for (int a = 0; a < 10; a++) {
// come out of loop when j is 5
if (a == 5) {
System.out.println("test break here");
break;
}
System.out.println("a: " + a);
}
System.out.println("After for loop");
while (b < 10) {
b += 2;
// come out of loop when b is greater than 4
if (b >= 5) {
System.out.println("test break here");
break;
}
System.out.println("b: " + b);
}
System.out.println("After while loop");
}
}
Output for the above program
a: 0
a: 1
a: 2
a: 3
a: 4
test break here
After for loop
b: 2
b: 4
test break here
After while loop
Let us hop to the next branching statement,continue statement in java.
The continue statement in java
This statement is used to skip a particular iteration statement and jump to the next iteration.
Example program to implement continue in java
class ContinueExp{
public static void main(String args[]) {
for (int i = 1; i < 15; i++) {
// If the number is even then bypass and continue with next iteration
if (i % 2 == 0)
continue;
// printing only odd numbers
System.out.print(i + " ");
}
}
}
Output for the above program
1 3 5 7 9 11 13
Let us hop to the next branching statement,return statement in java.
The return statement in java
The return statements are usually used at the end of the function or where you want to bypass the function. The return keyword is used to return the estimated value from the function to the calling function.
Example program to implement return in java
public class Main {
static int myMethod(int x) {
return 10 + x;
}
public static void main(String[] args) {
System.out.println(myMethod(13));
}
}
Output for the above program
23
This was all about control statements in java, their implementation and use cases along with example programs.To get in depth knowledge of core Java and advanced java, J2EE SOA training along with its various applications and real-time projects using Servlets, Spring with Aspect Oriented Programming (AOP) architecture, Hibernate Framework,and Struts through JDBC you can enroll in Certified Java Training in Chennai or Certified Java Training in Bangalore by FITA or a virtual class for this course,at an affordable price, bundled with real time projects, certification, support, and career guidance assistance and an active placement cell, to make you an industry required certified java developer.
FITA’s courses training is delivered by professional experts who have worked in the software development and testing industry for a minimum of 10+ years, and have experience of working with different software frameworks and software testing designs.