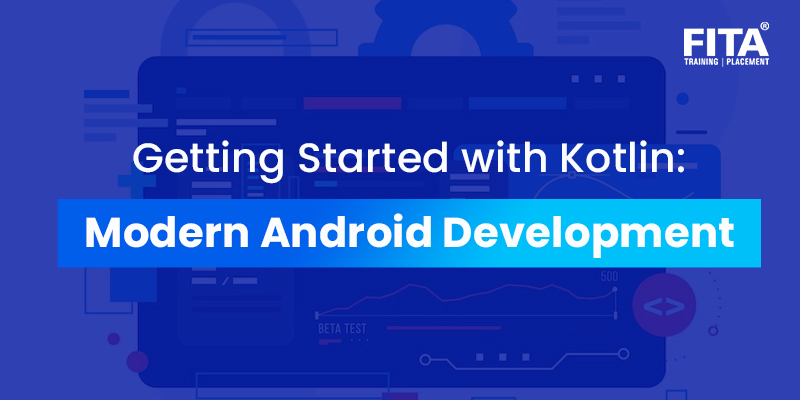
Android development has evolved rapidly over the years, and Kotlin has emerged as the preferred programming language for building modern Android applications. With its concise syntax, null safety, and seamless interoperability with existing Java code, Kotlin offers numerous advantages to developers. In this blog post, we will explore the essentials of getting started with Kotlin for modern Android development. From setting up the development environment to writing your first Kotlin application, this guide will equip you with the foundational knowledge to embark on your Android development journey.
Join Kotlin Android Developer Training in Chennai at FITA Academy and start learning Android development with the basics of the kotlin language.
Setting up the Development Environment
Before diving into Android app development with Kotlin, it’s crucial to have a proper development environment set up. This section will guide you through installing Android Studio, the official IDE for Android development, and configuring it for Kotlin projects. We will cover SDK installation, emulator setup, and creating a new Kotlin project.
Install Java Development Kit (JDK)
Kotlin runs on the JVM (Java Virtual Machine), so you’ll need to install the JDK to compile and run Kotlin code. Download and install the latest JDK version compatible with Kotlin from the Oracle or OpenJDK website.
Choose an Integrated Development Environment (IDE)
Kotlin is officially supported by JetBrains, the creators of IntelliJ IDEA. You can download IntelliJ IDEA, which has excellent Kotlin support out of the box. Alternatively, you can use other IDEs like Android Studio or Eclipse with Kotlin plugins.
Install Kotlin Plugin
If you’re using IntelliJ IDEA, you don’t need to install the Kotlin plugin separately, as it comes bundled with the IDE. For other IDEs, install the Kotlin plugin through the IDE’s plugin manager.
Build Tools
Kotlin projects can be built using popular build tools like Gradle or Maven. Make sure to install the corresponding build tool and configure it in your project.
Version Control
Set up a version control system like Git to track changes in your codebase. Install Git and configure it with your preferred Git client or IDE.
Dependencies and Package Management
Use a dependency management tools like Gradle or Maven to handle external libraries and dependencies in your Kotlin projects. Configure your build tool to manage and fetch dependencies automatically.
Testing Framework
Kotlin supports various testing frameworks such as JUnit and Spek. Configure your build tool to include the necessary testing dependencies and set up your preferred testing framework.
Code Quality Tools
Use static code analysis tools like detekt or ktlint to maintain code quality and enforce coding standards in your Kotlin projects. Integrate these tools into your build process or IDE to automatically analyse and format your code.
Continuous Integration (CI)
Set up a continuous integration server like Jenkins or Travis CI to automatically build, test, and deploy your Kotlin projects. Configure your CI server to execute the necessary build steps and tests upon code changes.
Enrol FITA Academy and be a part of Kotlin Certification Course in Bangaloreย and start building a career in Android development using kotlin.
Introduction to Kotlin Syntax
To start coding in Kotlin, understanding its syntax is essential. This section will introduce you to the fundamental Kotlin concepts, such as variables, data types, control flow statements, and functions. Additionally, you will explore Kotlin’s null safety feature, which helps prevent null pointer exceptions.
Sure! Kotlin is a statically typed programming coding language that load on the Java Virtual Machine (JVM) and can be used for various purposes, including Android app development, server-side development, and more. Its syntax is designed to be concise, expressive, and interoperable with existing Java code. Let’s explore the key aspects of Kotlin syntax professionally:
Variables and Data Types
- Variable Declaration: Kotlin provides the `val` and `var` keywords to declare read-only and mutable variables, respectively.
- Type Inference: Kotlin supports type inference, allowing you to omit explicit type declarations when the compiler can infer the type from the assigned value.
- Basic Data Types: Kotlin includes basic data types such as `Int`, `Double`, `Boolean`, `String`, etc., similar to Java. It also introduces nullable types with the `?` symbol, which helps prevent null pointer exceptions.
Control Flow
Conditional Statements: Kotlin provides the `if`, `else if`, and `else` constructs for conditional Looping Constructs: Kotlin supports `for` and `while` loops for iterating over collections or performing iterative tasks. Additionally, it provides the `break` and `continue` keywords for control flow manipulation within loops.
Functions
- Function Declaration: Kotlin uses the `fun` keyword to declare functions. It allows named parameters, default parameter values, and the ability to specify the return type explicitly or rely on type inference.
- Higher-Order Functions: Kotlin supports higher-order functions, which means Functions can be returned from other functions or supplied as parameters. This enables powerful functional programming capabilities.
Null Safety
- Nullability: Kotlin tackles the common issue of null pointer exceptions by incorporating null safety features. By default, variables cannot hold null values unless explicitly marked as nullable using the `?` symbol.
- Safe Calls: To safely access properties or call methods on nullable objects, Kotlin provides the safe call operator (`?.`), which returns `null` if the object is null instead of throwing an exception.
- Elvis Operator: The Elvis operator (`?:`) allows you to provide a default value when accessing a nullable object, which is used if the object is null.
Classes and Objects
- Class Declaration: Kotlin provides a concise syntax for declaring classes. The class body contains properties, methods, and initialiser blocks.
- Constructors: Kotlin introduces primary and secondary constructors. The primary constructor is declared in the class header, while secondary constructors are defined using the `constructor` keyword.
- Object Declaration: Kotlin supports the `object` keyword to define singleton objects, which are classes that have only one instance.
Extensions and Infix Functions
- Extension Functions: Kotlin allows you to extend existing classes with new functions using extension functions. These functions can be called as if they were regular member functions of the extended class.
- Infix Functions: Kotlin provides the `infix` keyword, which allows you to create infix functions that can be called using infix notation, omitting the dot and parentheses.
Smart Casts and Type Checks
- Smart Casts: Kotlin’s smart casts eliminate the need for explicit type casting in certain scenarios. When the compiler can guarantee that a variable is of a specific type, it automatically casts it.
- Type Checks: Kotlin provides the `is` operator for type checking. It allows you to check if an object is of a specific type and conditionally perform operations based on the result.
- These are some of the core aspects of android development using kotlin syntax. As you delve deeper into Kotlin development, you’ll encounter more advanced features and concepts that further enhance your coding experience.
Do you want to learn Android development? Join Android Training in Chennai and learn Android development from the basics with our experienced trainers.
Working with Kotlin and Java Interoperability
Kotlin provides seamless interoperability with existing Java codebases, enabling developers to leverage their existing Java knowledge and libraries. This section will explain how to call Java code from Kotlin and vice versa. You will also learn about Kotlin’s syntax enhancements and the benefits they bring when working with Java code.
Calling Java Code from Kotlin
Kotlin treats Java classes as first-class citizens, allowing you to directly use Java classes and call their methods.
- Importing Java Classes: Kotlin automatically imports Java classes from the same package, but for classes in different packages, you need to use the `import` statement explicitly.
- Handling Java Nullability: Kotlin’s null safety features apply to Java interop as well. Kotlin marks all Java references as potentially nullable, as Java doesn’t enforce null safety.
- Handling Java Overloaded Methods: When calling overloaded Java methods from Kotlin, you may need to disambiguate by specifying the parameter types explicitly.
Using Kotlin Libraries in Java
- Kotlin Standard Library: Kotlin provides an extensive standard library that can be used seamlessly in Java. Import the necessary Kotlin classes and use them in your Java code just like any other Java class.
- Nullable Types: Kotlin’s nullable types are represented in Java as `@Nullable` annotations. When using Kotlin libraries in Java, be aware of nullable types and handle them accordingly.
Interoperability Annotations
Kotlin offers a set of annotations specifically designed for Java interoperability. For example, `@JvmStatic` can be used to expose Kotlin functions or properties as static members in Java, and `@JvmOverloads` generates overloaded Java methods for Kotlin functions with default parameter values.
Nullable Types and Platform Types
Java lacks built-in nullability checks, but Kotlin provides null safety. When working with Java code that doesn’t specify nullability, Kotlin treats the corresponding types as platform types (`T!`), indicating that nullability is unknown. Exercise caution when working with platform types to avoid null pointer exceptions.
Using Java Libraries with Kotlin Features
- Kotlin provides several language features that can enhance working with Java libraries. For example, Kotlin’s extension functions allow you to add additional functionality to Java classes without modifying the source code.
- Consider leveraging Kotlin’s concise syntax, functional programming capabilities, and null safety features when working with Java libraries to improve code readability, maintainability, and safety.
Gradual Migration
If you’re migrating an existing Java codebase to Kotlin, consider adopting a gradual migration approach. Start by converting individual files or modules to Kotlin, ensuring that the Kotlin code integrates smoothly with the existing Java code. This allows for step-by-step adoption and minimises disruption.
Testing and Debugging
When working with Kotlin and Java code together, ensure that your testing and debugging processes cover both languages. Test the interoperability between Kotlin and Java components to identify and resolve any issues.
Continuous Integration
Configure your continuous integration (CI) system to build and test both Kotlin and Java code. Validate the interoperability between the two languages in your CI pipeline to catch any integration issues early on.
Documentation and Communication
Document the interoperability aspects of your Kotlin and Java codebase, especially if working in a team or collaborating with developers using different languages. Communicate the guidelines and best practices for working with Kotlin and Java to ensure consistent and effective collaboration.
By following these professional practices, you can effectively work with kotlin application development and Java interoperability, leverage the strengths of both languages and smoothly integrate Kotlin into existing Java projects.
Building User Interfaces with Kotlin
Building user interfaces with Android with Kotlin professionally involves leveraging frameworks like Android Jetpack or JavaFX for native or cross-platform app development. Utilise XML layouts or Kotlin DSLs to define UI elements, handle events, and manage data binding.
Employ best practices for UI design patterns, such as MVVM or MVP, to ensure the separation of concerns. Leverage Kotlin’s concise syntax, extension functions, and coroutines for asynchronous UI updates. Implement responsive and accessible UI designs, support internationalisation, and conduct thorough testing to ensure a polished user experience. Additionally, adhere to design guidelines, follow Material Design principles, and optimise performance for a professional UI development process.
Leveraging Kotlin Extensions
Leveraging Android with kotlin file extension professionally involves utilising the language’s extension functions and properties to enhance code readability, improve productivity, and enable fluent API designs. By extending existing classes, you can add new functionality without modifying their source code. Use extensions to encapsulate common operations, simplify complex tasks, or provide utility methods specific to your application domain. Follow best practices such as using specific naming conventions, documenting extensions, and keeping them focused and concise. Kotlin extensions enable clean and expressive code, facilitate code reuse, and contribute to a professional development process by promoting modular, maintainable, and efficient codebases.
Kotlin Coroutines: Simplifying Asynchronous Programming
Handling asynchronous operations is a common requirement in android development using kotlin. Kotlin Coroutines provide an elegant and efficient way to manage concurrency. In this section, you will learn about coroutines, their benefits, and how to use them to write clean and efficient asynchronous code.
Testing and Debugging Kotlin Applications
Writing tests and debugging are integral parts of the development process. This section will cover the testing and debugging tools available for Kotlin Android applications. How to create unit tests will be taught to you, run instrumented tests on Android devices, and debug your application using Android Studio’s powerful debugging features.
Join Android Training in Coimbatore and start learning the basics of android development and different frameworks
Deploying Kotlin Applications
Once you have completed kotlin application development and tested your Kotlin app, it’s time to deploy it to users. This section will guide you through the process of building a release-ready APK, signing it, and publishing it to the Google Play Store. We will cover the necessary steps to ensure your app is ready for production use.
Congratulations! You have completed the journey of getting started with Kotlin for modern Android development. This blog article included a summary of the essential concepts and tools to empower you to build robust and feature-rich Android applications using Kotlin.
Kotlin has revolutionised android with kotlin development by offering a powerful and expressive language. By following this guide and diving deeper into the world of Kotlin, you will unlock new possibilities for building modern Android applications with efficiency and ease.