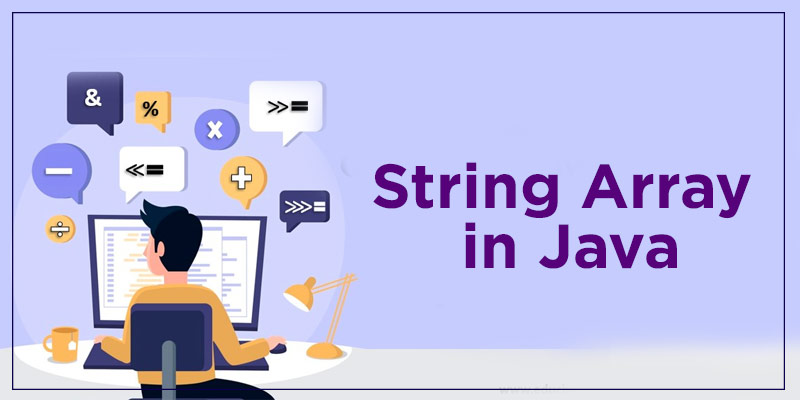
String Arrays in Java
We know that arrays in java are lists or collections of elements, strings in java behave similarly to arrays. They are character arrays and immutable, that is their size cannot be changed once defined. The characters are stored at contiguous memory locations just like arrays. Different operations such as searching for a character, sorting the string, splitting the string, or converting a string array as string iterating through characters, etc, can be performed on string arrays.
The main method public static void main(String[] args) ย of core java takes string arrays as arguments.
Let us see how to implement string arrays in java. Make sure you have java installed for implementation.Declaring String Array in Java
String array can be declared in 2 ways,// example program for declaring string Arrays in Java
import java.util.*;
class Main {
public static void main(String[] args) {
String[] strArr_1; // declaring without size
String[] strArr_2 = new String[2]; // declaring with size
}
}
If you print the array elements, you will get a null value for the size number of times, which is once for the first string array and twice for the second string array.
After learning how to declare String Arrays in Java, let us now learn how to Initialise String Arrays in Java.
Initialising String Array in Java
A string array can be initialised in 2ways; Example program for string array initialisation// example program for initialising string arrays in java
import java.util.*;
class Main {
public static void main(String[] args) {
// inline initialization
String[] strArr_1 = new String[] { "A", "E", "I", "O", "U" };
String[] strArr_2 = { "A", "E", "I", "O", "U" };
// initialization after declaration
String[] strArr_4 = new String[5];
strArr_4[0] = "A";
strArr_4[1] = "E";
strArr_4[2] = "I";
strArr_4[3] = "O";
strArr_4[4] = "U";
}
}
Accessing characters from the string array
Just like elements can be accessed from an array using an index, characters can also be accessed with the index or position of the character in the string array.
Here is an example program for accessing characters from a string array
// example program for accessing characters from the string array
import java.util.*;
class Main {
public static void main(String[] args) {
// inline initialization
String[] strArr_1 = new String[] { "A", "E", "I", "O", "U" };
String[] strArr_2 = { "A", "E", "I", "O", "U" };
System.out.println(strArr_1[0]); // first char from strArr_1
System.out.println(strArr_2[3]); // fourth char from strArr_2
System.out.println(strArr_1[4]);// last char from strArr_1
}
}
A
O
U
Here the strArr[0] is the first element in the array and strArr[4] is the last element in the array, which is also one less than the size of the array because the indexing starts at zero.
If you try to access strArr[5] or at any other index which is not present in the string array, it will throw at you an exception error of java.lang.ArrayIndexOutOfBoundsException
Check out this Complete Java Online Course by FITA Academy. FITA provides a complete Java course including core java and advanced java J2EE, and SOA training, where you will be building real-time applications using Servlets, Hibernate Framework, and Spring with Aspect Oriented Programming (AOP) architecture, Struts through JDBC bundled with, placement support, and certification at an affordable price with an active placement cell,by expert software developers with over 10 years of experience in the field to make you an industry required certified java developer.
After learning how to access String Arrays in Java, let us now learn how to find the size of String Arrays in Java.Size of String Array
The size of the string array is the number of elements or characters in the array, irrespective of the index of the last element. Well, the size or the length of an array or string array can be found using the length variable in java. For Example
// example program for finding the size of the string Array
import java.util.*;
class Main {
public static void main(String[] args) {
// Initializing string arrays
String[] strArr_1 = new String[] { "A", "E", "I", "O", "U" };
String[] strArr_2 = { "F","I","T","A"};
// printing length of the string using length variable
System.out.println(strArr_1.length);
System.out.println(strArr_2.length);
}
}
5
4
Traversing String Array
We can iterate through the string array and get each element at a time using a for loop, or for each loop.// example program for traversing through the string Array using for loop
import java.util.*;
class Main {
public static void main(String[] args) {
String[] strarr_1 = { "F", "I", "T", "A" };
String[] strarr_2 = { "FITA", "Academy" };
//iterating through all the characters in the array using for loop
for (int i = 0; i < strarr_1.length; i++) {
System.out.println(strarr_1[i]);
}
System.out.println();
for (int i = 0; i < strarr_2.length; i++) {
System.out.print(strarr_2[i]+" ");
}
}
}
F
I
T
A
FITA Academy
// example program for finding the size of the string Array using for each loop
import java.util.*;
class Main {
public static void main(String[] args) {
String[] strArr_1 = { "F", "I", "T", "A" };
String[] strArr_2 =new String[] { "FITA", "Academy" };
// iterating through all the characters in the array using foreach
for (String str : strArr_1) {
System.out.print(str+" ");
}
System.out.println();
for (String str : strArr_2) {
System.out.print(str+" ");
}
}
}
F I T A
FITA Academy
Searching for a value in String Array
We can search for a value in a string by looping over each element and comparing it with the value, this method will return the index of the first found match in the array, For example,
// example program for searching for a value from the string Array
import java.util.*;
public class Main {
public static void main(String[] args) {
String[] strArr = { "F", "I", "T", "A", "a", "c", "a", "d", "emy" };
Scanner scn = new Scanner(System.in);
boolean found = false;
int index = 0;
System.out.print("Find the index of ");
String str = scn.nextLine();
len = strArr.length
int pos = 0;
for (int pos; len> pos; pos++) {
if (str.equals(strArr[pos])) {
index = pos;
found = true;
break;
}
}
if (found)
System.out.print( " foundโ+str + in the string array at the index:"+ index);
else
System.out.print("Could not find " + str + "in the string array, str");
scn.close();
}
}
Find the index of A
A found at the index: 3
Find the index of a
a found at the index: 4
Converting string array to a string
The toString() method can be used on arrays to convert string array to string. Here is an example program// example program for converting string Array to a string using toString method
import java.util.*;
public class Main {
public static void main(String[] args) {
String[] strArr = { "F", "I", "T", "A", "a", "c", "a", "d", "emy" };
String theString = Arrays.toString(strArr);
System.out.println(theString);
}
}
[F, I, T, A, a, c, a, d, emy]
// example program for converting string Array to a string using StringBuilder class
import java.util.*;
public class Main {
public static void main(String[] args) {
String[] strArr = { "F", "I", "T", "A", "a", "c", "a", "d", "emy" };
String delimiter = " ";
StringBuilder sb = new StringBuilder();
for (String ch : strArr) {
if (sb.length() > 0) {
sb.append(delimiter);
}
sb.append(ch);
}
String theString = sb.toString();
System.out.println(theString);
}
}
F I T A a c a d emy
Sorting an array
We can arrange the string array alphabetically using the sort() method on the array.// example program for sorting the string Array
import java.util.*;
public class Main {
public static void main(String[] args) {
String[] strArr = { "F", "I", "T", "A", "a", "c", "a", "d", "emy" };
System.out.println("Initial string array: " + Arrays.toString(strArr));
Arrays.sort(strArr);
System.out.println("sorted string array: " + Arrays.toString(strArr));
}
}
Initial string array: [F, I, T, A, a, c, a, d, emy]
string array after sorting: [A, F, I, T, a, a, c, d, emy]
Converting A String Array To A List
Unlike arrays, a list is mutable, and its size can be changed. We can change the array to list using the asList() method. Here is an example for converting a string to list
// example program for converting string arrays to a list using asList method
import java.util.*;
public class Main {
public static void main(String[] args) {
String[] strArr = { "F", "I", "T","A" };
List<String> strLst = Arrays.asList(strArr);
System.out.print(strLst); // outputs [F,I,T,A]
}
}
// example program for finding the size of the string Array using ArrayList
import java.util.*;
public class Main {
public static void main(String[] args) {
String[] strArr = { "F", "I", "T","A" };
List<String> fxdLst = Arrays.asList(strArr);
List<String> strLst = new ArrayList<String>(fxdLst);
strLst.add("Academy");
System.out.print(strLst);
}
}
[F, I, T, A, Academy]
Converting String Array To A Set
A set is a data structure and an unordered collection of elements just like an array or list, except that it cannot contain duplicate elements. Letโs convert our previous array to a set using the HashSet class. Example program to demonstrate string set
// example program for converting string Array to a set
import java.util.*;
public class Main {
public static void main(String[] args) {
String[] strArr = { "F", "I", "T", "A","a","c","a","d","emy" };
List<String> strLst = Arrays.asList(strArr);
Set<String> strSt = new HashSet<String>(strLst);
System.out.println("Size of list: " + strLst.size());
System.out.println("Size of the set is: " + strSt.size());
System.out.print(strSt);
}
}
The size of the original list is: 9
The size of the set is: 8
[emy, A, a, c, T, d, F, I]
Converting List To A String Array
We have covered most of the operations on string arrays and now for the final one, we will convert a list to the string using the toArray() method. Here is how with an example,// example program for converting List to a String Array
import java.util.*;
public class Main {
public static void main(String[] args) {
//String[] strArr = { "F", "I", "T", "A", "a", "c", "a", "d", "emy" };
List<String> strLst = new ArrayList<String>();
strLst.add("F");
strLst.add("I");
strLst.add("T");
strLst.add("A");
strLst.add("Academy");
String[] strArr = strLst.toArray(new String[] {});
for (String ch : strArr) {
System.out.println(ch+" ");
}
}
}
F
I
T
A
Academy
There are many more string methods available, and I would recommend you to practice these problems on strings in java.
This was all about strings, arrays, and string arrays in java along with practice programs. To get in-depth knowledge of core Java and advanced java, J2EEย SOA training along with its various applications and real-time projects using Servlets, Spring with Aspect-Oriented Programming (AOP) architecture, Hibernate Framework, and Struts through JDBC you can enroll in Certified Java Training in Chennai or Certified Java Training in Bangalore by FITA or a virtual class for this course,at an affordable price, bundled with real-time projects, certification, support, and career guidance assistance and an active placement cell, to make you an industry required certified java developer.
FITAโs courses training is delivered by professional experts who have worked in the software development and testing industry for a minimum of 10+ years, and have experience of working with different software frameworks and software testing designs.
ย