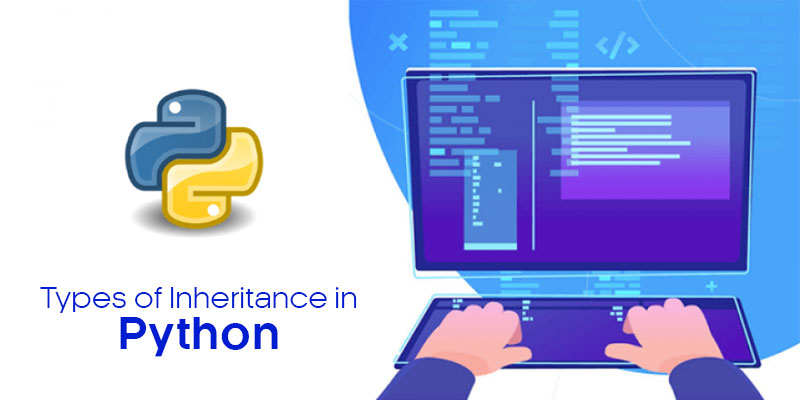
Let’s discuss the following things in this blog.
You might have some resemblance or inherited behaviours or traits in you from your family’s lineage. Just like this, you can inherit properties of one class from another one.The newly inherited class is a child or derived class and the former as parent or base class.Inheritance emphasizes the DRY(Don’t Repeat Yourself) rule, with code reusability.
Here is an example of Inheritance
class Parent:
def func1(self):
print(“I am in parent class”)
class Child(Parent):
def func2(self):
print(“I am in derived class”)
obj = Child()
obj.func1()
obj.func2()
Single Level Inheritance
Check out this Complete Online Python Course by FITA. FITA provides a complete Python course where you will be building real-time projects like Bitly and Twitter bundled with Django, placement support and certification at an affordable price.
In single level inheritance, there is only one child or derived class from a parent or base class.The example below is a one to one relation between base and a derived class.
class Article:
def __init__(self, title, content, author):
self.title = title
self.content = content
self.author = author
def represent(self):
return f'{self.author}is an author of
‘{self.title}’
Class Post(Article):
def __init__(self, title,content,author):
super().__init__(title,content,author)
art_1 = Article(‘Automate the Boring Stuff’,
‘Practical Programming..’,
‘AL Sweigart’)
art_2= Post(‘Python Tricks’,
‘A Buffet of Awesome Python..’,
‘Dan Bader’,’book.jpg’)
The __init__ method
You might have noticed the __init__ method in the above program. Such prefixed and postfixed double unscored methods are known as magic methods or dunder methods. This method is a constructor or initializer for attributes.A class recognizes its attributes with the self keyword.
The super() method
The super().__init__ method calls the constructor for the Base class with defined parent class attributes i.e., you can use parent class attributes and methods in the Base class.
Passing an argument of class name as base class to a derived class is known as subclassing.By this the derived class identifies its base class.
Multiple Inheritance
In multiple inheritance, a child class has more than one parent class.Here’s an example
class Father:
def func1(self):
print(“I am your dad!”)
class Mother:
def func2(self):
print(“I am your mom!”)
class Child(Father, Mother):
def func3(self):
print(“Hi mom and dad”)
ob = Child()
ob.func1()
ob.func2()
ob.func3()
Multilevel Inheritance
A Multi-level instance is when a derived class is a base class for another class. For instance , like this
class Father:
def func1(self):
print(“this is your father”)
class Son(Father):
def func2(self):
print(“this is your son”)
class GrandSon(Son):
def func3(self):
print(“this is your grandson”)
obj = GrandSon()
obj.func1()
obj.func2()
obj.func3()
Hierarchical Inheritance
Hierarchical Inheritance involves multiple inheritances from the same Parent or Child classes.
class Parent:
def func1(self):
print(“I am your Dad”)
class FirstSon(Parent):
def func2(self):
print(“I am the eldest brother”)
class SecondSon(Parent):
def func3(self):
print(“I am the youngest brother”)
obj1 = FirstSon()
obj2 = SecondSon()
obj1.func1()
obj2.func2()
Hybrid Inheritance
Hybrid Inheritance is when there is multiple and multilevel inheritance in a particular program.
class GrandParent:
def func1(self):
print(“I am Your grandpa!”)
class Father(GrandParent):
def func2(self):
print(“I am your father!”)
class Uncle(GrandParent):
def func3(self):
print(“I am your uncle!”):
class GrandSon(GrandParent , Father):
def func4(self):
print(“I am the youngest son.”)
obj = GrandSon()
obj.func1()
This was all about Inheritance and its types, applications and implementation in python.There is a lot more covered about this topic in Online Python Course. More over there is also an on campus version of this course as Python Training in Chennai or Python Training in Bangalore by FITA at an affordable price, which includes real time projects ,certification, support and career guidance assistance.