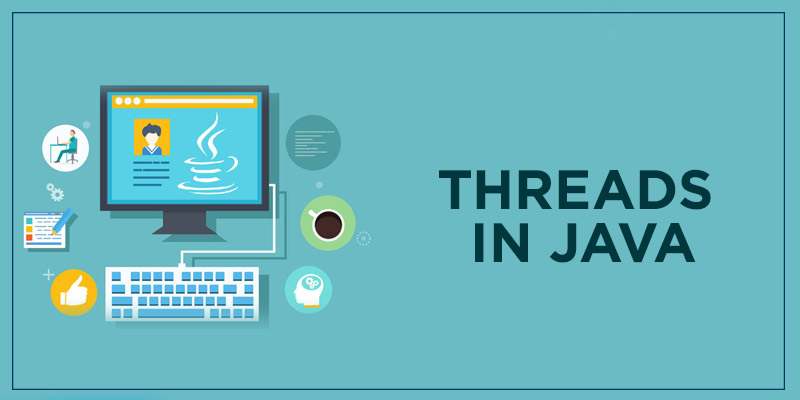
In this blog, we will dive deeper into understanding the Threads in Java, with these steps…
Let us now dive deep in to each of these topics one by one starting with..
What are Threads in Java?
When you are using your mobile, you might be watching a video or checking messages until the song downloads, or in a common sense you would be using multiple applications at the same time. This is known as multithreading, where each of the applications is running under a thread.
In java, a thread is a lightweight process or smallest independent unit of program which is created and controlled by the java.lang.Thread class.
If you understand well what is a thread in java, let’s move on to our next topic, Java Thread Life Cycle.
Java Thread Life Cycle
Whenever we create a thread, it will have a lifecycle which means it can lie only in one of the shown states at any point of time.Those states are
States Of A Thread
A new thread begins in this state and remains here until the program starts, therefore it is also known as a born thread.
Once a new thread starts, the tread comes under a runnable state where the program is ready to be run or is already running
In the waiting state, a thread is either temporarily inactive or has been blocked and the thread cannot move forward until it is again moved to the runnable state.For instance , when the program is waiting for an input or output to complete, it is under waiting thread.Moreover when a thread is in a blocked or waiting state, there might be any other thread running as scheduled by the thread scheduler.
The threads in waiting or blocked state do not consume any CPU cycle.
A thread enters a terminated state, when the running thread has completed its tasks, or if there is any unusual event like exceptions, segment fault or errors.
These were the states of a java thread, let us now move on to understanding the Main Thread Of Java.
Main Thread Of Java
By default we have a main thread in the java applications which is represented by the main method.The code in main method is executed by the main method in a sequence.So the main thread is created automatically when you run the program, and responsible for performing shut down operations as well.All the other child threads are dependent on the main thread.
Assigning all the tasks to the main method or main thread can make our program slow because of a long running process and can even show the “The program is not responding, do you want to wait or kill the program” dialog box.
This was about the main thread of Java.So let us understand how to create a separate thread in java using the Thread class and the Runnable Interface.
Creating A Thread In Java
Java has a built in support for creating threads, unlike many other programming languages.
A thread in java can be created in two ways:
Check out this Complete Java Training Online by FITA. FITA provides a complete Java course including core java and advanced java J2EE, and SOA training, where you will be building real time applications using Servlets, Hibernate Framework, and Spring with Aspect Oriented Programming (AOP) architecture, Struts through JDBC bundled with, placement support, and certification at an affordable price with an active placement cell, to make you an industry required certified java developer.
Let us now understand how to create a thread using each of these methods with example programs in java.
Creating threads with Thread class
import java.io.*;
public class Images extends Thread {
public void run() {
System.out.println("The Images app is running");
}
public static void main(String[] args) {
Images trd1 = new Images();
trd1.start();
}
}
Output for the above program
The Images app is running
Hope you understood creating a single with the Thread class, and now let us see how to create a thread with a runnable interface.
Runnable Interface in Java
In most of the cases your class will be already inheriting any other class , and at the same time to implement the Thread class on it, you cannot inherit the Thread class on the super class, since multiple inheritance is not supported by java,so instead we can use the runnable interface to implement threading.
So creating thread using runnable interface requires the following steps
Here is an example of creating a thread in java by extending the Thread class to our custom class
import java.io.*;
public class Videos implements Runnable {
public void run() {
System.out.println("The Videos app is running");
}
public static void main(String[] args) {
Videos trd2 = new Videos();
trd2.start();
}
}
Output for the above program
The videos app is running
Let us understand what is multi threading in Java and how to implement it.
Multi threading in java
So far we have been creating a single thread, and using the 2 threads in a program (main and custom thread). However a program can have more than 2 or multiple threads running each after the other or with a wait in between multiple threads.
There are many methods which helps managing the threads, few of them are:
SYNTAX for getName
public String getName():
SYNTAX for the getPriority method
public final int getPriority()
SYNTAX for the isAlive method
public final boolean isAlive()
SYNTAX for the join method
public final void join()
SYNTAX for calling the run method
object.start()
SYNTAX for the sleep method
public static void sleep(long m, int n)
SYNTAX for start
public void start()
Let me show you an example for creating and running multiple threads in an application.
import java.io.*;
// Java code for running multiple threads in an application
class Multithreads implements Runnable
{
public void run()
{
try
{
// Printing the thread which is running
System.out.println ("Running Thread #" +
Thread.currentThread().getId());
for (int j=0; j<5;j++){
System.out.println ("Do this under Thread " +
Thread.currentThread().getName());
}
Thread.sleep(1000);
}
catch (Exception e)
{
// Throwing an exception
System.out.println ("An Exception is caught while running multiple threads");
}
}
}
// Main Class
class Mainthread
{
public static void main(String[] args)
{
int n = 10; // Number of threads
for (int i=0; i<n; i++)
{
Thread trdObj = new Thread(new Multithreads());
trdObj.start();
}
}
}
Output for the above program
Running Thread #13
Running Thread #18
Running Thread #19
Running Thread #16
Running Thread #20
Running Thread #14
Running Thread #15
Running Thread #11
Do this under ThreadThread-7
Do this under ThreadThread-7
Do this under ThreadThread-7
Do this under ThreadThread-7
Do this under ThreadThread-7
Do this under ThreadThread-5
Do this under ThreadThread-5
Do this under ThreadThread-5
Do this under ThreadThread-5
Do this under ThreadThread-5
Do this under ThreadThread-9
Running Thread #17
Running Thread #12
Do this under ThreadThread-1
Do this under ThreadThread-1
Do this under ThreadThread-1
Do this under ThreadThread-1
Do this under ThreadThread-1
Do this under ThreadThread-4
Do this under ThreadThread-4
Do this under ThreadThread-4
Do this under ThreadThread-4
Do this under ThreadThread-4
Do this under ThreadThread-6
Do this under ThreadThread-6
Do this under ThreadThread-6
Do this under ThreadThread-6
Do this under ThreadThread-6
Do this under ThreadThread-8
Do this under ThreadThread-2
Do this under ThreadThread-3
Do this under ThreadThread-9
Do this under ThreadThread-0
Do this under ThreadThread-9
Do this under ThreadThread-3
Do this under ThreadThread-8
Do this under ThreadThread-2
Do this under ThreadThread-3
Do this under ThreadThread-9
Do this under ThreadThread-0
Do this under ThreadThread-9
Do this under ThreadThread-3
Do this under ThreadThread-8
Do this under ThreadThread-2
Do this under ThreadThread-3
Do this under ThreadThread-0
Do this under ThreadThread-0
Do this under ThreadThread-0
Do this under ThreadThread-8
Do this under ThreadThread-2
Do this under ThreadThread-2
Do this under ThreadThread-8
This was all about threads, creating a thread with Runnable Interface and Thread class and multithreading in java along with example programs for implementation.
To get in depth knowledge of core Java and advanced java, J2EE along with its various applications and real-time projects using Servlets, Spring with Aspect Oriented Programming (AOP) architecture, Hibernate Framework,and Struts through JDBC you can enroll in Java Training in Chennai or Java Training in Bangalore by FITA or a virtual class for these courses at an affordable price, bundled with real time projects, certification, support, and career guidance assistance with an active placement cell, to make you an industry required certified java developer.
FITA’s courses training is delivered by professional experts who have worked in the software development and testing industry for a minimum of 10+ years, and have experience of working with different software frameworks and software testing designs.