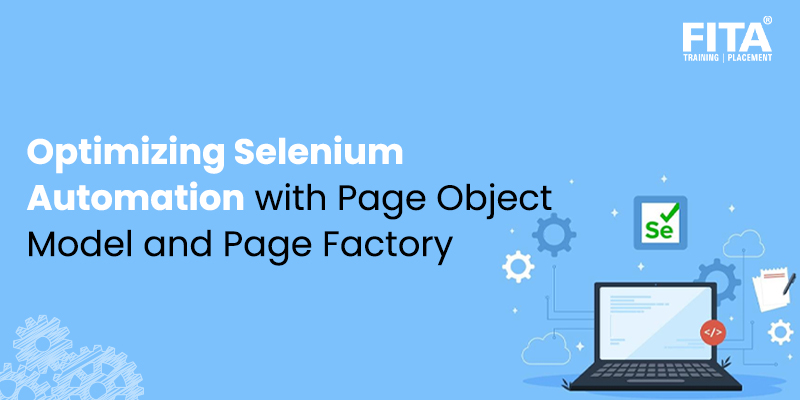
Creating automated tests is not merely a convenience but an indispensable asset for any agile software development team. It serves as a crucial instrument for swiftly identifying defects in the early stages of the software development life cycle. When a new feature is still in its developmental phase, developers can execute automated tests to evaluate the impact of these modifications on other components of the system. This article will elucidate the process of expediting this procedure through test automation in Selenium, employing the Page Object model.
Test automation offers the potential to reduce the expenses associated with rectifying defects while enhancing the overall quality assurance (QA) process for software. By implementing comprehensive tests, developers can pinpoint and resolve defects prior to their escalation to the QA phase. Test automation also streamlines the automation of test cases and consistently regressing features. Consequently, QA engineers gain more time to scrutinize other facets of the application, thereby fortifying the product’s quality during production releases. To delve deeper into test automation tools like Selenium and enhance your skill set, consider enrolling in Selenium Training in Chennai.
Selenium Page Object Model
The Page Object model is a design pattern in Selenium that treats webpages as classes, with various page elements defined as variables within those classes. All possible user interactions are then implemented as methods within these classes. This approach provides an elegant way to create test routines that are both readable and easily maintainable or updatable in the future. For instance:
To support the Page Object model, we employ Page Factory. Page Factory in Selenium extends the Page Object pattern and offers various usage options. In this case, we will use Page Factory to initialise web elements defined in web page classes or Page Objects.
To make use of web element classes or Page Objects containing web elements, they must be initialised using the Page Factory before the web element variables can be utilised. This is accomplished using the `initElements` function within Page Factory:
LoginPage page = new LoginPage(driver);
PageFactory.initElements(driver, page);
Alternatively, a more concise initialisation can be achieved as follows:
LoginPage page = PageFactory.initElements(driver, LoginPage.class);
Another approach is to initialise elements inside the webpage class constructor:
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
Page Factory initialises each WebElement variable with a reference to the corresponding element on the actual webpage based on configured “locators.” This configuration is done through the use of `@FindBy` annotations, allowing us to define a strategy for locating the element, along with the necessary information to identify it:
@FindBy(how = How.NAME, using = "username")
private WebElement user_name;
Every time a method is called on this WebElement variable, the driver will first locate it on the current page and then simulate the interaction. If we are working with a simple page where the element will be found each time we search for it, and we navigate away from the page without returning, we can cache the looked-up field using the `@CacheLookup` annotation:
@FindBy(how = How.NAME, using = "username")
@CacheLookup
private WebElement user_name;
This entire WebElement variable definition can be simplified as:
@FindBy(name = "username")
private WebElement user_name;
The `@FindBy` annotation supports various other strategies, such as id, name, className, CSS, tagName, linkText, partialLinkText, and xpath.
Once initialised, these WebElement variables can be used to interact with the corresponding elements on the page. For instance, we can input a sequence of keystrokes into the password field on the page:
user_password.sendKeys(password);
This is equivalent to the following:
driver.findElement(By.name("user_password")).sendKeys(password);
Additionally, you may encounter situations where you need to locate a list of elements on a page, and that’s when `@FindBys` becomes useful:
@FindBys(@FindBy(css = "div[class='yt-lockup-tile yt-lockup-video']"))
private List videoElements;
This code finds all `div` elements with two class names, `yt-lockup-tile` and `yt-lockup-video`. We can simplify this further:
@FindBy(how = How.CSS, using = "div[class='yt-lockup-tile yt-lockup-video']")
private List videoElements;
You can also use `@FindAll` with multiple `@FindBy` annotations to look for elements that match any of the provided locators:
@FindAll({ @FindBy(how = How.ID, using = "username"), @FindBy(className = "username-field") })
private WebElement user_name;
Now that we can represent webpages as Java classes and easily initialise WebElement variables using Page Factory, let’s explore how to write simple tests using the Page Object pattern and Page Factory in Selenium.
Simple Selenium Test Automation Project in Java
To execute this automation, we need to set up our Java project. Here are the general steps to get started:
- Install Java: If you haven’t already, you’ll need to install Java on your system. You can download and install the Java Development Kit (JDK) from the official Oracle website.
- Install an Integrated Development Environment (IDE): You can choose from various IDEs for Java development. Two popular options are Eclipse and IntelliJ IDEA. Download and install your preferred IDE.
- Set up a Selenium WebDriver: You’ll need to download the Selenium WebDriver for Java. WebDriver is a tool for automating web applications, and it provides APIs for various programming languages, including Java.
- Create a Java Project: Open your IDE and create a new Java project for your automation testing.
- Configure Selenium Dependencies: In your Java project, configure the Selenium WebDriver dependencies. You can use a build automation tool like Maven or Gradle to manage your project dependencies. Add the Selenium WebDriver and any other required libraries to your project’s build path. To become a selenium tester, check out Selenium Interview Questions and Answers, it has various types of interview questions and tips.
- Create Page Objects: Implement the Page Object model by creating Java classes that represent webpages and define web elements and methods to interact with them. Each page of the fita sign-up process should have its own Page Object class.
- Write Test Scripts: Create test scripts using Java and Selenium WebDriver to automate the steps you outlined earlier. Your test scripts should use the Page Objects you created to interact with the web elements on the fita website.
- Execute Tests: Run your test scripts to automate the fita developer sign-up process. You can execute tests from your IDE or using a testing framework like JUnit or TestNG.
- Generate Reports: Consider using a reporting tool like ExtentReports or TestNG reports to generate test execution reports for better visibility into test results.
- Review and Debug: Continuously review your code and test results. Debug any issues that arise during test execution.
- Refine and Maintain: As the fita website or your test requirements change, update your Page Objects and test scripts accordingly to ensure your automation remains accurate and reliable.
Remember to handle waits appropriately in your test scripts to ensure that elements on the webpage are fully loaded before interacting with them. This will help prevent flakiness in your automated tests.
By following these steps and best practices for test automation, you can successfully automate the fita developer sign-up process using Selenium’s Page Object model with Java.
Setting Up a Project
Certainly, I’ll provide you with step-by-step instructions on setting up a Maven project in IntelliJ IDEA for Selenium testing. Follow these steps:
1. Install Java JDK and IntelliJ IDEA
Download and install the Java JDK from the official Oracle website:
[Java SE Downloads] (https://www.oracle.com/java/technologies/javase-downloads.html) .Download](https://www.jetbrains.com/idea/download/).
2. Create a New Maven Project
- Open IntelliJ IDEA.
- Click on “File” > “New” > “Project…”
- In the “New Project” dialog, select “Maven” on the left panel.
- Choose “Create from archetype” and select “maven-archetype-quickstart.”
- Click “Next.”
3. Configure Project Settings
- Set the “GroupId” to something like “SeleniumTEST” (you can choose your own).
- Set the “ArtifactId” to “Test” (or any name you prefer).
- Choose a location for your project.
- Click “Next.”
4. Configure JDK
- In the “Project SDK” dropdown, select the Java JDK you installed earlier. For example, on Windows, it might be located at “C:\Program Files\Java\jdkxxx.”
- Click “Next” and then “Finish.”
5. Update the POM (Project Object Model) File
In the “pom.xml” file, which is part of your project, add the following dependencies for Selenium and JUnit Maven. Make sure to update the `selenium.version` and `junit.version` with the latest version numbers:
junit
junit
${junit.version}
test
org.seleniumhq.selenium
selenium-firefox-driver
${selenium.version}
org.seleniumhq.selenium
selenium-support
${selenium.version}
org.seleniumhq.selenium
selenium-java
${selenium.version}
6. Enable Auto-Build and Download Dependencies
If auto-build is enabled, dependencies should start downloading automatically. If not, activate it by going to “Plugins” > “install” > “install:install” under the “Maven Projects” panel on the right side of your IntelliJ IDEA IDE.
7. Create Package Structure
In the “src/test/java” directory, create a package named `com.fita`.
Within the `com.fita` package, create two more packages: `com.fita.webpages` and `com.fita.tests`. Your package structure should look like this:
src
└── test
└── java
└── com
└── fita
├── webpages
└── tests
Now, you have set up a Maven project in IntelliJ IDEA with the necessary dependencies for Selenium testing. You can proceed to create your Page Object/Page Factory classes under the `com.fita.webpages` package and test routines under the `com.fita.tests` package. Join Selenium Training in Pune and start learning about selenium from the basics from professionals.
Selenium Page Object Model: HomePage
Your `HomePage` class for fita’s homepage looks well-structured and follows the Page Object pattern. It initialises web elements and provides methods for interacting with those elements. Here’s a brief overview of the class:
package com.fita.webpages;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.How;
import org.openqa.selenium.support.PageFactory;
public class HomePage {
private WebDriver driver;
// Page URL
private static String PAGE_URL = "https://www.fita.com";
// Locators
// Apply as Developer Button
@FindBy(how = How.LINK_TEXT, using = "APPLY AS A DEVELOPER")
private WebElement developerApplyButton;
// Constructor
public HomePage(WebDriver driver) {
this.driver = driver;
driver.get(PAGE_URL);
// Initialize Elements
PageFactory.initElements(driver, this);
}
// Method to click on the "APPLY AS A DEVELOPER" button
public void clickOnDeveloperApplyButton() {
developerApplyButton.click();
}
}
This class effectively represents the fita homepage. It initializes the web elements, such as the “APPLY AS A DEVELOPER” button, and provides a method `clickOnDeveloperApplyButton()` to click on that button. This structured approach will make your test code cleaner and more maintainable when interacting with the fita homepage in your Selenium tests. You can similarly create Page Object classes for other pages in your application.
Are you from Pondicherry? Looking for a standard affordable training centre? Check out our Selenium Training in Pondicherry today itself!
Writing a Simple Selenium Test
Your `ApplyAsDeveloperTest` class appears to be well-structured, following the Page Object pattern and making use of WebDriver for Selenium automation. Here’s an overview of your test routine:
package com.fita.tests;
import com.fita.webpages.DeveloperApplyPage;
import com.fita.webpages.DeveloperPortalPage;
import com.fita.webpages.HomePage;
import org.junit.After;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import java.util.concurrent.TimeUnit;
public class ApplyAsDeveloperTest {
WebDriver driver;
@Before
public void setup() {
// Use Firefox Driver
driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
}
@Test
public void applyAsDeveloper() {
// Create an object of HomePage Class
HomePage home = new HomePage(driver);
home.clickOnDeveloperApplyButton();
// Create an object of DeveloperPortalPage
DeveloperPortalPage devportal = new DeveloperPortalPage(driver);
// Check if the page is opened
Assert.assertTrue(devportal.isPageOpened());
// Click on Join fita
devportal.clickOnJoin();
// Create an object of DeveloperApplyPage
DeveloperApplyPage applyPage = new DeveloperApplyPage(driver);
// Check if the page is opened
Assert.assertTrue(applyPage.isPageOpened());
// Fill in data
applyPage.setDeveloper_email("dejan@fita.com");
applyPage.setDeveloper_full_name("Dejan Zivanovic Automated Test");
applyPage.setDeveloper_password("password123");
applyPage.setDeveloper_password_confirmation("password123");
applyPage.setDeveloper_skype("automated_test_skype");
// Click on join
// applyPage.clickOnJoin();
}
@After
public void close() {
driver.close();
}
}
This test class sets up the WebDriver, interacts with the fita homepage, developer portal page, and developer apply page using Page Objects, and includes assertions to check if the expected pages are opened. It also contains the `@Before` and `@After` methods for test setup and cleanup, respectively.
To complete your test, you can uncomment the `applyPage.clickOnJoin();` line to simulate clicking the “Join” button on the apply page once you’ve filled in the required data. Make sure that your Page Object methods and locators are correctly implemented for this to work as expected.
With this structure, your test cases will be more organised and maintainable, and you can easily extend them to cover more scenarios as needed. Enrol for Selenium Training in Bangalore and be a pro in selenium testing!
Automation in Selenium for Maintainable Test Suites
Page Object and Page Factory in Selenium streamline the process of modeling and automating web page testing, greatly simplifying the tasks of developers and QA engineers. When implemented correctly, these Page Object classes can be leveraged throughout your entire test suite, allowing for the early integration of automated Selenium tests into your projects without disrupting agile development practices to become a selenium tester. By encapsulating user interactions within page object models and maintaining lightweight and straightforward test routines, you can effortlessly adapt your test suite to accommodate evolving requirements.