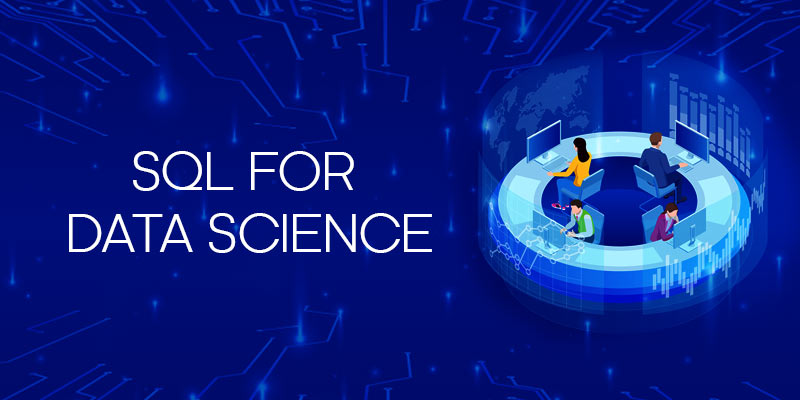
Data has become the fuel for the organizations to strategize and formulate the entire models as per trending patterns and requirements. Databases are where these data reside, but working with them requires a programming language.
SQL or Structured Query Language is a primary language for working with databases. It is used widely for data analysis or data science, it is a must to know the language for driving, reports, manipulation, or analysis of a dataset.
With SQL you can update, add or delete records from the database.The top Relational Database Management Systems(RDBMS) such as PostgreSQL, MySql, SQLite, MariaDB, Oracle are based on the SQL.
This blog is your way to go for analyzing simple datasets using SQL.
Here is what we will discuss step by step in this blog, Let us now learn the Sql starting with the Basics Of SQL…Basic Of SQL
Creating Database A SQL Database is a godown for storing structured data. Here is how you would create a database in MySQL.
-- syntax for creating a database
CREATE DATABASE <databasename>
USE <databasename>
-- Example for creating a database
CREATE DATABASE FITA
USE FITA
-- syntax for creating a table
CREATE TABLE <tablename>
(
variable1 datatype 1,
variable 2 datatype 2,
variable n datatype n,
..
);
Here the CREATE TABLE will create a table with a table name. The variables here are the name of the attributes, which will be the columns of the table.SQL data can have numeric, character or string Date and Time, Binary, and Boolean data type. A semicolon indicates the termination.
-- Example for creating a table
CREATE TABLE Students
(
roll number INTEGER PRIMARY KEY AUTOINCREMENT NOT NULL,
student_name TEXT,
marks INTEGER,
grade VARCHAR,
passed BOOLEAN
);
Here the roll number is of type integer and the primary key is for identifying records with this unique value. There could be only one primary key for a record.
AUTOINCREMENT is used to increment the value automatically, that is you wonโt need to pass the value for the roll number and it will be automatically set.
After creating the tables in the database let us now see how to insert rows in the table.
Insert into table
To insert a record into the table you will need to specify all the values with the respective data type in the table.
-- Syntax for inserting a record
INSERT INTO TABLE <tablename> VALUES
(
value 1,
value 2,
..
value n
);
INSERT INTO Students VALUES (NULL,โAtufaโ,70,โBโ,true);
INSERT INTO Students VALUES (NULL,โPujaโ,85,โAโ,true);
INSERT INTO Students VALUES (NULL,โJaneโ,50,โBโ,true);
INSERT INTO Students VALUES (NULL,โRosyโ,45,โCโ,true);
INSERT INTO Students VALUES (NULL,โHarryโ,20,โFโ,false);
Retrieving data
-- Example for selecting all the records
SELECT * FROM Students
roll_number | student_name | marks | grade | passed |
1 | Atufa | 70 | B | 1 |
2 | Puja | 85 | A | 1 |
3 | Jane | 50 | B | 1 |
4 | Rosy | 45 | C | 1 |
5 | Harry | 20 | F | 0 |
--ย Example for selecting records for specific attributes
SELECT student_name, passed FROM STUDENTS;
student_name | passed |
Puja | 1 |
Jane | 1 |
Rosy | 1 |
Harry | 0 |
SELECT * FROM Students WHERE grade == โBโ;
roll_number | student_name | marks | grade | passed |
1 | Atufa | 70 | B | 1 |
3 | Jane | 50 | B | 1 |
SELECT student_name FROM Students WHERE grade == โAโ;
student_name |
Puja |
SELECT student_name FROM Students WHERE marks>=35;
student_name |
Atufa |
Puja |
Jane |
Rosy |
SELECT * FROM Students WHERE marks>=35 ORDER BY grade;
Output for the above query
roll_number | student_name | marks | grade | passed |
2 | Puja | 85 | A | 1 |
1 | Atufa | 70 | B | 1 |
3 | Jane | 50 | B | 1 |
4 | Rosy | 45 | C | 1 |
Updating records from Table
To update records from the table you will simply need to use the UPDATE and SET command. Here are some examplesUPDATE Students SET grade = 'A' WHERE marks>=70;
SELECT marks, grade FROM Students;
marks | grade |
70 | A |
85 | A |
50 | B |
45 | C |
20 | F |
Check out this completeย Online Data Science Course by FITA, which includes Supervised, Unsupervised machine learning algorithms, Data Analysis Manipulation and visualization, reinforcement testing, hypothesis testing, and much more to make an industry required data scientist at an affordable price, which includes certification, support with career guidance assistance ย with an active placement cell,to make you an industry required certified data scientist.
After learning to update the records from the database let us now see how to count the records from the table in the database. Counting records The count() function can be used to count. Here are some examplesSELECT COUNT(*) FROM Students; -- outputs 5
SELECT COUNT(*) FROM Students WHERE marks>=35; -- outputs 4
DELETE FROM Students WHERE grade == 'F';
SELECT * FROM Students;
roll_number | student_name | marks | grade | passed |
1 | Atufa | 70 | A | 1 |
2 | Puja | 85 | A | 1 |
3 | Jane | 50 | B | 1 |
4 | Rosy | 45 | C | 1 |
DELETE FROM Students;
Deleting Table
To delete a table from the database using the DROP command with the table name. Here is the command to delete the table for students.DROP TABLE Students;
ADVANCED SQL QUERIES
Let us now check some of the advanced sql queries, for summing, filtering, and limiting the data. Aggregation Functions in SQL Here we will use the Students tables with the previous 5 records.SELECT SUM(marks) FROM Students; -- outputs 270
ECT AVG(marks) FROM Students WHERE marks>=35; -- outputs 62.5SEL
SELECT student_name,MAX(marks) FROM Students WHERE grade =='B';
student_name | max(marks) |
Jane | 50 |
Check out thisย SQL Training in Chennai. FITA provides a complete SQL course that covers all the beginning and the advanced queries of SQL.
Additionally it covers all the elements of ANSI SQL, Data Definition Language (DDL) and Data Manipulation Language (DML) using both SQL Server and Oracle, with an active placement cell,to make a certified Database Professional.
After learning the aggregate functions of SQL, for summing up the data, finding the minimum or maximum value from the data or the average let us now learn to filter the records from the database.
Filtering the data
Filters in SQL include WHERE, ORDER BY, BETWEEN, LIKE, GROUP BY, HAVING. We have already covered ORDER BY and WHERE. Letโs take a look at others. This statement usually works with aggregate functions (MAX, MIN, SUM, COUNT, AVG) to create sets of the same values. Here is an exampleSELECT COUNT(marks), grade FROM Students GROUP BY grade;
count(marks) | grade |
2 | A |
1 | B |
1 | C |
SELECT * FROM Students WHERE student_name LIKE '%Harry';
SELECT student_name, grade FROM Students WHERE marks BETWEEN 50 AND 75;
student_name | grade |
Atufa | A |
Jane | B |
SELECT grade, COUNT(*) FROM Students GROUP BY grade WHERE marks>35;
SELECT grade, COUNT(*) FROM Students GROUP BY grade HAVING marks>35;
grade | count(*) |
A | 2 |
B | 1 |
C | 1 |
Limiting the data
SELECT * FROM Students LIMIT 3;
SELECT * FROM Students LIMIT 3 OFFSET 2;
roll_number | student_name | marks | grade | passed |
3 | Jane | 50 | B | 1 |
4 | Rosy | 45 | C | 1 |
This was a quick start tutorial for SQL and its implementations. To get the in depth knowledge of Python along with its various applications and real-time projects, you can enroll inย Python Course in Chennai or Python Training in Bangaloreย or enroll for a Data science course at Chennai or Data science course in Bangalore or in virtual classes for these courses, which includes Supervised, Unsupervised machine learning algorithms, Data Analysis Manipulation and visualization, reinforcement testing, hypothesis testingย and much more with an active placement cell, to make an industry required data scientist at an affordable price, which includes certification, support with career guidance assistance to make you an industry required certified python developer and a certified data scientist.
FITAโs courses training is delivered by professional experts who have worked in the software development and testing industry for a minimum of 10+ years, and have experience of working with different software frameworks and software testing designs.