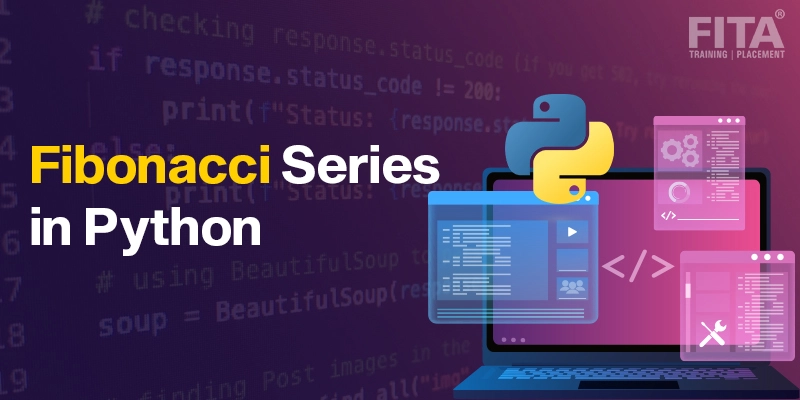
The Fibonacci series is one of the most fascinating sequences in mathematics, appearing in nature, art, and even financial markets. In programming, it’s a great way to understand recursion, loops, and efficiency in algorithms. Whether you’re a beginner learning Python or an experienced developer exploring algorithm optimization, implementing the Fibonacci sequence is a fundamental exercise.
In this blog, we’ll break down multiple ways to generate the Fibonacci series in Python, from simple recursion to dynamic programming, helping you write efficient and scalable code. Firstly,
What is Fibonacci Sequence?
The Fibonacci numbers sequence is a series of numbers where each number is the total sum of the two preceding numbers that start from 0 and 1.
The sequence follows this pattern:
Fibonacci Sequence:
list of fibonacci numbers: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, …
Fibonacci sequence follows a pattern where each number is the sum of the two preceding ones, starting from 0 and 1. That is, Mathematically, the nth term in Fibonacci numbers series is represented as is represented as: F(n)=F(n−1)+F(n−2)F(n) = F(n-1) + F(n-2) where:
- F(0)=0F(0) = 0
- F(1)=1F(1) = 1
How to write a program of fibonacci series?
Now, let’s explore different methods to implement the Fibonacci sequence in Python.
1. Fibonacci Series in Python Using Recursion
# Function to generate Fibonacci series using recursion
def fib(num):
if num <= 1:
return num
else:
return fib(num - 1) + fib(num - 2)
nu = int(input("Enter the number of terms you want: "))
if nu <= 0:
print("Give a natural number")
else:
for n in range(nu):
print(fib(n), end=", ")
Output:
Enter the number of terms you want: 4
0, 1, 1, 2,
Steps Involved:
- Define a function to return Fibonacci numbers.
- If the input is 1, return 0.
- If the input is 2, return 1.
- Otherwise, recursively call the function for (n−1)+(n−2)(n – 1) + (n – 2).
“Checkout our blog How to Convert List to String in Python?, to learn more”
2. Fibonacci Series in Python Using Dynamic Programming
# Function to generate Fibonacci series using Dynamic Programming
def fibonacci_dp(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_dp(n-1, memo) + fibonacci_dp(n-2, memo)
return memo[n]
num = int(input("Enter the number of terms: "))
for i in range(num):
print(fibonacci_dp(i), end=", ")
Steps Involved:
- Initialize a dictionary to store Fibonacci numbers series.
- Check if the number already exists in the dictionary.
- If not, compute and store it in the dictionary.
- Use recursion with memoization to avoid redundant calculations.
“Eager to explore the diverse roles Python plays within Google? Check out our blog on Roles of Python programming language in Google today.”
Turn Your Creativity into a Career!
Learn Data Analytics at FITA Academy from expert trainers.
Explore Program3. Fibonacci Series in Python Using While Loop
# Function to generate Fibonacci series using While Loop
n1, n2 = 0, 1
count = 0
num = int(input("Enter the number of terms: "))
if num <= 0:
print("Please enter a positive number")
elif num == 1:
print("Fibonacci sequence:", n1)
else:
print("Fibonacci sequence:")
while count < num:
print(n1, end=", ")
nth = n1 + n2
n1, n2 = n2, nth
count += 1
Steps Involved:
- Take input from the user.
- Initialize first two terms (n1 = 0, n2 = 1) and count = 0.
- If the input is more than 2, use a while loop to calculate terms.
- Add previous two numbers to get the next term.
- Swap values and keep incrementing the count until the limit is reached.
“Check out our latest blog on Python Interview Questions and Answers to ace your job interviews with expert insights, real-world examples, and detailed explanations.”
4. Fibonacci Series in C vs. Python
If you are wondering about fibonacci series in C, here’s how it looks:
#include
void fibonacci(int n) {
int a = 0, b = 1, c;
for (int i = 0; i < n; i++) {
printf("%d, ", a);
c = a + b;
a = b;
b = c;
}
}
int main() {
int num;
printf("Enter the number of terms: ");
scanf("%d", &num);
fibonacci(num);
return 0;
}
“Wondering how to write a program to generate a Fibonacci series? We got you! Checkout our blog Goto Statement in Python, to learn Python code for the Fibonacci sequence.”
Fibonacci series in Python vs Fibonacci Series in C
Feature | Fibonacci series in Python | Fibonacci Series in C |
Simplicity | ✅ Easy to implement with fewer lines of code | ❌ More complex with explicit type declarations |
Execution Speed | ❌ Slower for large fibonacci numbers series calculations | ✅ Faster execution for computing extensive fibonacci numbers series |
Readability | ✅ High readability with clean syntax | ❌ Lower readability with more syntax requirements |
Memory Management | ✅ Automatic (better for beginners) | ❌ Manual (better for optimization) |
Large Number Handling | ✅ Native support for arbitrary precision integers | ❌ Requires special libraries for very large fibonacci numbers |
Wondering which is better for implementing the Fibonacci series: If you want computational speed when generating the Fibonacci numbers series, use C. If you want simplicity and readability when implementing fibonacci series, use Python.
“And if you are looking to enhance your understanding about Fibonacci sequence code python, enroll in FITA Academy’s Python Training in Bangalore.”
What Fibonacci Code is Used For?
Many people ask, what Fibonacci code is used for? The Fibonacci series is applied in Computer Science: Search algorithms (Fibonacci search), Stock Market Analysis: Fibonacci retracements in trading, Nature: Growth patterns in plants, shells, and galaxies.
Frequently Asked Questions about Fibonacci Series
1. What Fibonacci series used for?
The Fibonacci number series is widely used in computer algorithms, financial market analysis, nature (e.g., the arrangement of leaves, flowers, and shells), and even in data science for pattern recognition.
2. How does the Fibonacci sequence series relate to the Golden Ratio?
As the Fibonacci sequence series progresses, the ratio of two consecutive numbers becomes the Golden Ratio (approximately 1.618), which appears in many natural and artistic structures.
The demand for data-driven professionals is skyrocketing.
Learn Data Analytics at FITA Academy and fit yourself into the demand.
Explore Program3. Can Fibonacci be used in real-world applications?
Yes! The Fibonacci series number is used in:
- Stock market analysis
- Computer algorithms (e.g., Fibonacci search technique)
- Cryptography
- Biology and nature (e.g., branching in trees, shells, flowers)
4. Why is recursion not the best approach for Fibonacci numbers series?
Recursion leads to a high number of redundant calculations, making it inefficient for large values. Using Dynamic Programming or Iteration is a better approach as they significantly reduce computation time.
5. What is the time complexity of different Fibonacci implementations?
- Recursion: O(2^n) (Exponential Time Complexity)
- Dynamic Programming (Memoization): O(n) (Linear Time Complexity)
- Iterative Approach (While Loop): O(n) (Linear Time Complexity)
6. How to generate a Fibonacci series in C?
The Fibonacci series in C can be generated using recursion, loops, or arrays, similar to Python implementations.
7. What is the difference between Fibonacci numbers and Fibonacci sequence?
Fibonacci numbers refer to individual terms in the Fibonacci series, while the Fibonacci sequence refers to the ordered list of these numbers.
8. Is the Fibonacci sequence series infinite?
Yes, the Fibonacci sequence series continues indefinitely as each term is derived from the last two terms.
9. Can Fibonacci number series be negative?
Negative Fibonacci series can be defined using a variation called Negafibonacci, where terms are generated using the formula.
10. What is the largest Fibonacci number that can fit in an integer?
The largest Fibonacci number series that fit in a 32-bit sized integer is 2,971,215,073, corresponding to F(47).
11. What are some real-life patterns related to the Fibonacci sequence?
The Fibonacci sequence appears in natural phenomena like sunflower seed arrangements, pine cones, tree branching, and human anatomy proportions.
12. Can Fibonacci be used in AI and machine learning?
Yes! Fibonacci-based algorithms can be used in neural network optimizations, data structure analysis, and genetic algorithms.
Boost Your Career with Cutting-Edge IT Courses through a Master Program at FITA Academy!
Enquire Now13. How can we optimize the Fibonacci numbers series generation?
The Fibonacci series can be optimized using:
- Memoization (Dynamic Programming)
- Matrix Exponentiation
- Binet’s Formula (for direct calculation)
14. What is Binet’s formula for Fibonacci numbers series?
Binet’s formula is where (the golden ratio) is approximately 1.618.
15. Is there a connection between Fibonacci and Pascal’s Triangle?
Yes! The sum of diagonals in Pascal’s Triangle follows the Fibonacci sequence.
To get in-depth knowledge of the Fibonacci series in Python, the fibonacci series coding along with its various applications and real-time projects, you can enroll in Python Training in Chennai by FITA Academy at an affordable price, which includes certification, support with career guidance assistance.