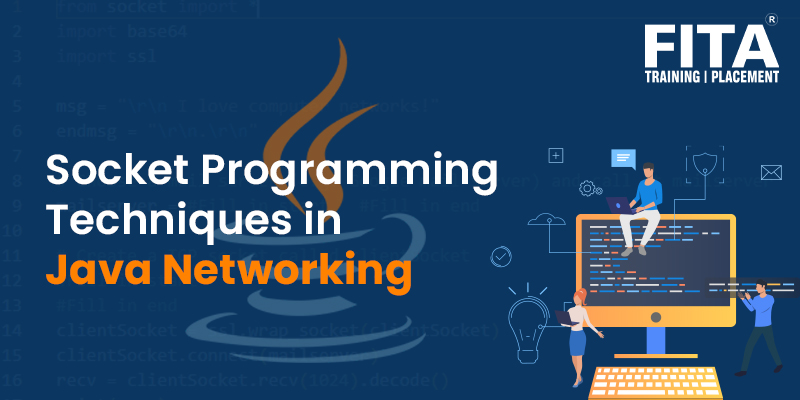
Networking refers to the communication and connection of computing devices, which can include a diverse range of devices such as laptops, desktops, servers, smartphones, tablets, and an ever-growing array of Internet of Things (IoT) gadgets. In a networked environment, these devices share information and data with each other, facilitating communication and collaboration.
The concept of networking extends beyond traditional computing devices to encompass a wide range of IoT devices, which includes cameras, door locks, doorbells, refrigerators, audio/visual systems, thermostats, and various sensors. These devices are interconnected to enable seamless data exchange and communication, contributing to the creation of smart and interconnected systems.
Networking plays a crucial role in enabling the flow of information and facilitating the integration of devices and systems. It forms the backbone of modern communication infrastructure, allowing individuals and organizations to access and share resources, communicate, and collaborate efficiently. Networking technologies can include wired and wireless connections, protocols, and standards that govern the exchange of data between devices, ensuring reliable and secure communication.
The significance of networking has grown exponentially with the rise of the Internet and the proliferation of connected devices. Whether in local area networks (LANs), wide area networks (WANs), or the global Internet, networking technologies have become fundamental to our daily lives, enabling the seamless flow of information and supporting the functionality of numerous applications and services. For those looking to delve into the world of software development and enhance their programming skills, considering a specialized program like Java Training in Chennai can provide a solid foundation in one of the most widely used programming languages in the industry.
What is Java Networking?
Networking empowers even simple programs with significant capabilities, allowing a single program to access information stored on millions of computers worldwide. Java, as a programming language, stands out in this context, being designed from the ground up with networking in mind. Java Networking involves the integration of two or more computing devices to facilitate the sharing of resources.
In Java, all program communications over a network occur at the application layer. The Java platform provides the java.net package within the J2SE APIs, which includes a variety of classes and interfaces. These components handle the low-level aspects of communication, empowering developers to create java programs that effectively address networking challenges.
The java.net package offers a robust set of tools for network programming in Java. It includes classes for handling sockets, URLs, and other network-related operations. Sockets, for instance, enable communication between programs running on different devices over a network. URLs facilitate the handling of web addresses and resources. These tools, combined with the object-oriented features of Java, simplify the process of developing networked applications.
Java’s networking capabilities find extensive use in various domains, including web development, distributed systems, and enterprise applications. The language’s design principles prioritize platform independence, making Java applications highly adaptable to different environments. As a result, Java has become a preferred choice for developing networked applications where portability, reliability, and ease of development are crucial.
Java Networking empowers Java developers to create applications that can seamlessly communicate and share resources across diverse computing devices connected through networks, contributing to the robustness and versatility of Java-based solutions. For individuals eager to master Java and harness its networking capabilities, exploring specialized training programs like Java Training in Coimbatore can provide hands-on experience and comprehensive knowledge in leveraging Java for effective networked applications.
Common Network Protocols
The java.net package in the Java programming language not only encompasses various classes and interfaces but also provides support for two fundamental network protocols: Transmission Control Protocol (TCP) and User Datagram Protocol (UDP).
Transmission Control Protocol (TCP)
TCP is a connection-oriented protocol designed for secure communication between different applications.
Connection-Oriented: TCP operates in a connection-oriented manner, meaning that once a connection is established between two devices, data can be transmitted bidirectionally.
Usage with IP: Typically used over the Internet Protocol (IP), TCP is often referred to as TCP/IP.
Reliability Features: TCP includes built-in methods to check for errors and ensure the ordered delivery of data. This makes it a comprehensive protocol suitable for transporting various types of information, such as still images, data files, and web pages.
User Datagram Protocol (UDP)
UDP is a connectionless protocol that allows the transmission of data packets between different applications.
Connectionless Nature: UDP operates in a connectionless manner, where there is no need for establishing, maintaining, or terminating a connection.
No Error-Checking Overhead: Unlike TCP, UDP does not provide error-checking and recovery services. It is a simpler protocol.
Continuous Data Transmission: In UDP, data is continually sent to the recipient, regardless of whether they receive it or not. This makes UDP suitable for scenarios where real-time communication is crucial and a slight loss of data is acceptable.
Java’s java.net package supports both TCP and UDP protocols, offering developers flexible options based on their specific networking requirements. TCP is favored for applications requiring reliable, ordered data transmission, while UDP is suitable for scenarios where a lightweight, connectionless approach with minimal overhead is preferred, often seen in real-time applications.
Java Network Topology
IP Address
- An IP address is a unique identifier for a device on the internet or a local network, governed by Internet Protocol rules.
- Its format are Comprising octets, each ranging from 0 to 255 (e.g., 192.168.0.1).
- It serves as a logical address, distinguishing devices in a network.
Port Number
- A numeric identifier for processes connecting to the internet, facilitating communication between applications.
- Rangesfrom 0 to 65,535, with specific ports assigned to distinct applications.
- It enables unique identification of applications for data transmission.
Protocol
- A set of rules governing data transmission between devices in a network.
- Its roles are standardized in the way data is communicated.
- Some examples are TCP (Transmission Control Protocol), FTP (File Transfer Protocol), POP (Post Office Protocol), etc.
MAC Address
- Media Access Control address, a unique identifier assigned to a Network Interface Controller (NIC).
- Its structure are A 48 or 64-bit hexadecimal address associated with the network adapter.
- It is Purpose for Used for device tracking in a network.
Socket
- An endpoint in a two-way communication connection between applications on a network.
- Facilitates Inter-Process Communication (IPC) by establishing contact points for communication.
- Tied to a port number, enabling data transmission to the intended application.
Connection-oriented protocol
Requires establishing a connection before communication, ensuring reliability.
Example: Transmission Control Protocol (TCP).
Connection-less protocol
Data transported without verifying continuous connectivity.
Example: User Datagram Protocol (UDP).
Authentication: Not required for connectionless protocols.
Understanding these terminologies is crucial for developing effective Java networking applications, enabling seamless communication and data exchange in networked environments.
Java Networking Classes
The java.net package in the Java programming language encompasses various classes facilitating easy access to network resources. Here are some key classes within this package:
CacheRequest
It isused for storing resources in ResponseCache, allowing OutputStream objects to store resource data into the cache.
CookieHandler
Implements a callback mechanism for setting up an HTTP state management policy within the HTTP protocol handler. Manages HTTP requests and responses.
CookieManager
It provides a precise implementation of CookieHandler, separating cookie storage from the policy of accepting and rejecting cookies. Comprises a CookieStore and a CookiePolicy.
DatagramPacket
Facilitates connectionless message transfer between systems. Enables the creation of datagram packets for connectionless transmission using the DatagramSocket class.
InetAddress
It provides methods to obtain the IP address of any hostname, handling both IPv4 and IPv6 addresses.
ServerSocket
It implements a system-independent server-side of a client/server socket connection. Throws an exception if it cannot listen on the specified port.
Socket
It creates socket objects for fundamental socket operations, enabling users to implement various networking actions such as sending, reading data, and closing connections.
DatagramSocket
A network socket for connection-less point-to-point communication. Provides the ability to send and receive packets independently, supporting broadcast information.
Proxy
It represents a tool or system that acts as an intermediary between computers and internet users, preserving user and computer data.
URL
It Describes a Uniform Resource Locator, serving as an entry point to any available sources on the internet.
URLConnection
An abstract class describing a connection to a resource defined by a URL. Allows control over interaction with a server, verification of headers, and configuration of header fields for client requests. These classes form the foundation for Java networking, providing developers with essential tools for network communication and resource access.
Java Networking Interfaces
The java.net package in the Java programming language not only includes various classes but also comprises essential interfaces that facilitate easy access to network resources. Here are some key interfaces within this package:
CookiePolicy
Allows implementation of various networking applications. Decides which cookies should be accepted or rejected. Pre-defined policies include ACCEPT_ALL, ACCEPT_NONE, and ACCEPT_ORIGINAL_SERVER.
CookieStore
Describes a storage space for cookies. CookieManager combines cookies to the CookieStore for each HTTP response and retrieves cookies from the CookieStore for each HTTP request.
FileNameMap
It provides a simple interface for defining a file name and a MIME type string. Charges a filename map (mimetable) from a data file.
SocketOption
It enables users to control the behavior of sockets. Essential for developing necessary features in sockets, allowing users to set various standard options.
SocketImplFactory
Defines a factory for SocketImpl instances. Used by the Socket class to create socket implementations that implement various policies.
ProtocolFamily
It Represents a family of communication protocols. Contains a method called name(), which returns the name of the protocol family.
These interfaces complement the classes in the java.net package, providing developers with a versatile set of tools for network communication and resource access. They play a crucial role in implementing networking applications and customizing the behavior of network-related features.
Socket Programming
Java Socket programming serves as a mechanism for communication between applications running on different Java Runtime Environments (JRE). It enables communication between two computers using TCP, and it can be either connection-oriented or connectionless. In connection-oriented socket programming, the Socket and ServerSocket classes are employed, while connectionless socket programming uses the DatagramSocket and DatagramPacket classes. For those looking to broaden their skill set beyond Java networking and delve into the field of data science, considering specialized training programs like Data Science Training in Bangalore can provide a solid foundation in analytics and insights derived from large datasets.
Here are the steps involved in establishing a TCP connection between two computers using Socket Programming:
Server Instantiation
The server creates a ServerSocket object, specifying the port number for communication.
Acceptance of Client Connection
The server calls the accept() method of the ServerSocket class, causing the program to wait until a client connects to the server on the specified port.
Client Instantiation
A client creates a Socket object, providing the server name and port number to connect to.
Connection Establishment
The Socket class constructor attempts to connect the client to the designated server and port. If successful, the client now has a Socket object capable of interacting with the server.
Server-Side Handling
On the server side, the accept() method returns a reference to a new socket connected to the client’s socket.
After the connection is established, communication occurs using Input/Output (I/O) streams. Each Socket object has both an OutputStream and an InputStream. The client’s OutputStream is linked to the server’s InputStream, and vice versa. TCP, being a two-way communication protocol, allows data transmission over both streams simultaneously.
Socket programming in Java provides a robust foundation for building networked applications, facilitating communication and data exchange between distributed systems. It is a fundamental aspect of creating networked applications ranging from simple client-server models to more complex distributed systems.
Socket Class
The Socket class in Java provides essential functionality for implementing socket operations, enabling users to perform various networking actions such as sending, reading data, and closing connections. Each Socket object created using the java.net.Socket class is specifically associated with one remote host. If a user intends to connect to another host, a new Socket object must be created.
Here are some important methods of the Socket class:
public void connect(SocketAddress host, int timeout)
This method is used to connect the socket to the specified host. It is required when the user instantiates the Socket using the no-argument constructor.
public int getPort()
Returns the port to which the socket is connected on the remote machine.
public InetAddress getInetAddress()
Returns the IP address of the other computer to which the socket is connected.
public int getLocalPort()
Returns the port to which the socket is bound on the local machine.
public SocketAddress getRemoteSocketAddress()
Returns the address of the remote socket.
public InputStream getInputStream()
Returns the input stream of the socket. This input stream is connected to the output stream of the remote socket.
public OutputStream getOutputStream()
Returns the output stream of the socket. This output stream is connected to the input stream of the remote socket.
public void close()
Closes the socket, rendering the Socket object unable to connect again to any server.
These methods provide the necessary functionality for managing socket connections, obtaining information about the socket, and handling input and output streams for communication between client and server applications.
ServerSocket Class
The ServerSocket class in Java is essential for providing a system-independent implementation of the server-side of a client/server socket connection. It allows the server to listen for incoming client connections on a specified port. Below are some important methods of the ServerSocket class:
public int getLocalPort()
Returns the port number to which the server socket is bound.
public void setSoTimeout(int timeout)
Sets the timeout value for the accept() method, which determines how long the server socket will wait for a client connection.
public Socket accept()
Waits for an incoming client connection. This method is blocked until a client connects to the server on the specified port or until a timeout occurs.
public void bind(SocketAddress host, int backlog)
Binds the server socket to a specified address and port. This method is used when the ServerSocket is instantiated using the no-argument constructor.
Here’s an example of a basic one-way client-server setup using Java socket programming:
Client-Side Java Implementation
import java.io.*;
import java.net.;
public class ClientSide {
private Socket socket = null;
private DataInputStream input = null;
private DataOutputStream out = null;
public ClientSide(String address, int port) {
try {
socket = new Socket(address, port);
System.out.println("Connected");
input = new DataInputStream(System.in);
out = new DataOutputStream(socket.getOutputStream());
} catch (UnknownHostException u) {
System.out.println(u);
} catch (IOException i) {
System.out.println(i);
}
String line = "";
while (!line.equals("End")) {
try {
line = input.readLine();
out.writeUTF(line);
} catch (IOException i) {
System.out.println(i);
}
}
try {
input.close();
out.close();
socket.close();
} catch (IOException i) {
System.out.println(i);
}
}
public static void main(String[] args) {
ClientSide client = new ClientSide("127.0.0.1", 5000);
}
}
Server Side Java Implementation
import java.io.*;
import java.net.*;
public class ServerSide {
private Socket socket = null;
private ServerSocket server = null;
private DataInputStream in = null;
public ServerSide(int port) {
try {
server = new ServerSocket(port);
System.out.println("Server started");
System.out.println("Waiting for a client ...");
socket = server.accept();
System.out.println("Client accepted");
in = new DataInputStream(new BufferedInputStream(socket.getInputStream()));
String line = "";
while (!line.equals("End")) {
try {
line = in.readUTF();
System.out.println(line);
} catch (IOException i) {
System.out.println(i);
}
}
System.out.println("Closing connection");
socket.close();
in.close();
} catch (IOException i) {
System.out.println(i);
}
}
public static void main(String[] args) {
ServerSide server = new ServerSide(5000);
}
}
This example demonstrates a basic client-server interaction where the client connects, sends messages to the server, and the server displays them. The communication is done using a socket connection. For individuals interested in expanding their programming expertise, exploring diverse applications, and gaining proficiency in a versatile language, considering a comprehensive program such as a Python Course in Coimbatore can offer valuable insights and hands-on experience in Python, a language known for its simplicity and versatility in various domains.
Inet Address
The InetAddress class in Java is crucial for obtaining the IP address of a given hostname. It provides methods to work with IP addresses, and it can handle both IPv4 and IPv6 addresses. An object of the InetAddress class represents an IP address and its corresponding hostname.
There are two main types of addresses that InetAddress can represent:
Unicast
- An identifier for a single interface.
- Refers to a unique address assigned to a specific network interface on a host.
Multicast
- An identifier for a collection of interfaces.
- Refers to an address that can be used by multiple hosts (interfaces) in a group for communication.
Here’s a simple explanation of how to use InetAddress in Java:
import java.net.InetAddress;
import java.net.UnknownHostException;
public class InetAddressExample {
public static void main(String[] args) {
try {
// Get the local host address
InetAddress localHost = InetAddress.getLocalHost();
System.out.println("Local Host: " + localHost);
// Get IP address by hostname
InetAddress googleAddress = InetAddress.getByName("www.google.com");
System.out.println("Google IP Address: " + googleAddress.getHostAddress());
// Get all IP addresses associated with a hostname
InetAddress[] allGoogleAddresses = InetAddress.getAllByName("www.google.com");
System.out.println("All Google IP Addresses:");
for (InetAddress address : allGoogleAddresses) {
System.out.println(address.getHostAddress());
}
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
In this example:
- The getLocalHost() method retrieves the local host’s InetAddress.
- The getByName(“www.google.com”) method gets the InetAddress associated with the specified hostname.
- The getAllByName(“www.google.com”) method returns an array of InetAddress objects, representing all IP addresses associated with the specified hostname.
- Keep in mind that handling exceptions, such as UnknownHostException, is important when working with network-related operations.
URL Class
The URL class in Java is a fundamental class for working with Uniform Resource Locators (URLs), which are addresses used to identify and locate resources on the internet. A URL typically consists of several components, each serving a specific purpose.
Components of URL
Here’s an overview of the components of a URL:
Protocol
- The protocol in a URL defines how information is transported between the host (server) and the client (or web browser).
- Examples of protocols include HTTP (Hypertext Transfer Protocol), HTTPS (HTTP Secure), FTP (File Transfer Protocol), etc.
Hostname
- The hostname is the name of the device (server) on which the resource exists.
- It is often represented by a domain name (e.g., www.example.com) or an IP address.
File Name
- The filename represents the pathname to the file or resource on the server.
- For web pages, this might include the path to the specific page or resource on the server.
Port Number
- The port number is used to identify different applications uniquely on the host.
- It is typically optional and is specified if the resource is served on a port other than the default for the given protocol.
Here’s a simple example of using the URL class in Java:
import java.net.URL;
public class URLExample {
public static void main(String[] args) {
try {
// Creating a URL object
URL url = new URL("https://www.example.com/path/to/resource");
// Retrieving components of the URL
String protocol = url.getProtocol();
String hostname = url.getHost();
String path = url.getPath();
int port = url.getPort(); // Returns -1 if not specified
// Displaying the components
System.out.println("Protocol: " + protocol);
System.out.println("Hostname: " + hostname);
System.out.println("Path: " + path);
System.out.println("Port: " + port);
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, the URL class is used to parse a URL string, and then its methods are employed to retrieve various components such as protocol, hostname, path, and port. Handling exceptions, such as MalformedURLException, is important when working with URLs. If you’re interested in expanding your technical skills, consider exploring relevant courses like a CCNA Course in Pondicherry, which can provide valuable insights into networking and connectivity aspects within the realm of information technology.